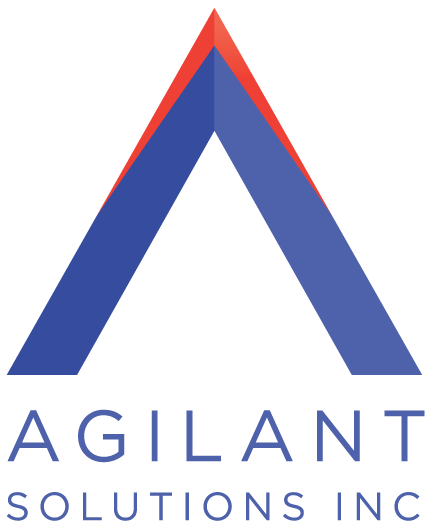
Overview
Introduction
This API implements functionality for utilizing the ticketing features of Agilant’s TOGaDesk system through a RESTful HTTP transaction containing a JSON payload.
Endpoints
Beta/Test/Sandbox Environmenthttps://api.beta.agilantsolutions.com
Production Environmenthttps://api.agilantsolutions.com
Structure & Schema
- All requests and responses are formatted in JSON notation.
- Any whitespace in the request payload is disregarded. The JSON examples in this document are shown in a "pretty print" structure for easier legibility but JSON is not required to be in "pretty print" when performing the actual transactions.
- This API is intended for businesses-to-businesses (B2B) and must be implemented on your server-side. Requests to this API should never be sent from or processed by a user on the client-side.
- There are no data or structural differences between our beta and production environment. The only change you must make to initiate a request in production is that it must be sent to our production URL endpoint.
- All parameter names are case-sensitive and all values are handled with case sensitivity.
- Connection timeouts should be set to 15 seconds.
- Date and times in any inputs and outputs are formatted in ISO 8601 notation.
- For example:
1994-11-05
corresponds to November 5, 1994. - For example:
1994-11-05T08:15:30-05:00
corresponds to November 5, 1994, 8:15:30 am, US Eastern Standard Time. - For example:
08:15:30-05:00
corresponds to 8:15:30 am, US Eastern Standard Time.
Authentication & Authorization
- During the onboarding process, Agilant will provide you with two GUID keys which you are to store. One key is a public API key and the other key is a secret API key. You must ensure that the secret key is not disclosed anywhere in your code to the public.
- In the header of every transaction, you will need to provide a timestamp, your public key, and a signature of your transaction comprising of the timestamp field signed by your secret API key using the HMAC-SHA-256 cryptographic hash algorithm.
- The timestamp must be within 120 seconds (2 minutes) of the actual time or your transaction will be rejected. Therefore, you must ensure that your system time is maintained accurately within 2 minutes of the actual universal time.
Required Request Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Must be set to application/json |
Timestamp | String | The current timestamp of your transaction in ISO 8601 notation. Example: 2019-01-02T17:34:52-05:00 |
Authorization | String | Concatenated string of your public API key, followed by a literal period (. ), followed by your signature. Your signature is your timestamp value, signed using your secret API key through the HMAC-SHA-256 cryptographic hash algorithm in a raw binary format and then Base64 encoded.Format: {publicKey}.{signatureOfTimestampUsingSecretKey} |
Sample Authentication Request Headers
Content-Type: application/json
Timestamp: 2019-01-02T17:34:52-05:00
Authorization: 1111AAAA-22BB-33CC-44DD-555555EEEEEE.Omjruf/UNd+rKEbjobxJzky84h6XOE9o9jz6/IKqc7Q=
Sample Authentication Request Headers
See the sample request using the sample information below.
- Using Sample Timestamp of:
2019-01-02T17:34:52-05:00
- Using Sample Public API Key of:
1111AAAA-22BB-33CC-44DD-555555EEEEEE
- Using Sample Secret API Key of:
EEEE5555-DD44-CC33-BB22-AAAAAA111111
Sample PHP Code for Assembling Request Headers
<?php
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
?>
Responses
- All responses include two fields called
success
anderror
. - The
success
field is a boolean data type which will returntrue
orfalse
for whether or not the transaction was successful. - The
error
field will contain an intelligible string of the error details if one occurred or will be an empty value if no error occurred. - Additionally, all transactions will respond with the following codes if they are successful:
200 OK
for allGET
,PUT
, andDELETE
requests.201 CREATED
for allPOST
requests.- When determining for whether or not the transaction was successful, you should check that the HTTP response code is
200 OK
or201 CREATED
and/or check that thesuccess
value is set totrue
.
Sample Response Headers
Content-Type: application/json
Content-Length: 354
Response Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Will always be set to application/json |
Content-Length | Integer | Length of the response payload in bytes. |
Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
401 UNAUTHORIZED | Authentication or authorization failed. | Review the returned errors and correct the issue. If the problem persists, you may not be permitted to perform this API call. |
403 FORBIDDEN | Access was denied when trying to retrieve data for a record. | Ensure you have proper authorization to access the record. |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
405 METHOD NOT ALLOWED | The requested action is not supported. | Ensure that you are calling a valid API method and using the correct method and route. |
408 REQUEST TIMEOUT | A connection error was encountered and the request may or may not have completed successfully. | Retry the transaction and/or perform other GET requests to check if the previous request succeeded. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
500 INTERNAL SERVER ERRROR | An unexpected internal server error was encountered. | Contact the administrator. Further analysis and debugging may need to be performed. |
502 BAD GATEWAY | A temporary network issue occurred when receiving the request. This is rare and typically caused by a very temporary network issue which should resolve within a few seconds. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
504 GATEWAY TIMEOUT | A network issue occurred when receiving the request. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
PHP Example
<?php
$endpoint = 'https://api.beta.agilantsolutions.com'; // SANDBOX ENDPOINT
//$endpoint = 'https://api.agilantsolutions.com'; // PRODUCTION ENDPOINT
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$method = 'GET';
$route = '/provider';
$payload = '{"providers":["C638C170-3EC1-4DF2-942C-1D1C3AD74FBC","16527A9E-4048-452CA8DF-605E1038A313"]}';
//////////////////////////////////////////////////
$url = ($endpoint . $route);
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, $method);
curl_setopt($ch, CURLOPT_POSTFIELDS, $payload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$responseBody = curl_exec($ch);
$responseCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
echo 'responseCode=' . $responseCode . '<br>';
echo 'responseBody=' . $responseBody;
///////////////////////////////////////////////
/*
EXAMPLE OUTPUT:
responseCode=200
responseBody=
{
"success": true,
"error": "",
"providers": [
{
"providerId": "C638C170-3EC1-4DF2-942C-1D1C3AD74FBC",
"providerName": "Porch",
"avgRating": 4.3,
"reviews": [
{
"name": "Jane Doe",
"rating": 4.7,
"review": "I like this service very much!"
},
{
"name": "John Smith",
"rating": 3.5,
"review": "I was quite happy!"
}
]
},
{
"providerId": "16527A9E-4048-452C-A8DF-605E1038A313",
"providerName": "Hello Tech",
"avgRating": 3.1,
"reviews": [
{
"name": "Mary Doe",
"rating": 2.7,
"review": "I was not happy with this service.
},
{
"name": "Jim Dean",
"rating": 4.1,
"review": "This looks really nice!"
}
]
}
]
}
*/
Enumerated Lists
Webhook Types
Defines the type of a webhook request
Values
Value | Description |
---|---|
TICKET_STATUS_CHANGE | When a ticket status has changed. |
TICKET_REPLY | When a ticket reply has been added. |
Ticket Statuses
Defines the status of a ticket
Values
Value | Description |
---|---|
NEW_TICKET | The ticket is newly submitted and has not been handled yet. |
OPEN | The ticket is open and is waiting for review. |
IN_PROGRESS | The ticket is in progress and is being handled. |
AWAITING_USER | The ticket is open and is awaiting the user to provide more information. |
CLOSED | The ticket has been closed. |
REVIEW | The ticket was closed and is now under review after being requested to re-open. |
Ticket Priorities
Defines the priority of a ticket
Values
Value | Description |
---|---|
LOW | A low-priority ticket. |
NORMAL | A normal-priority ticket. |
HIGH | A high-priority ticket. |
Data Objects
Ticket
Sample Ticket Object
{
"ticketNumber" : 12345678,
"priority" : "NORMAL",
"status" : "IN_PROGRESS",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "johnsmith@testco.com",
"phoneNumber" : "(123) 456-7890",
"subject" : "My computer won't boot up",
"message" : "I cannot get the computer to boot into Windows",
"replies" : [
{
"repliedOn" : "2020-02-03T07:34:16-06:00",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "janedoe@testco.com",
"message" : "Have you tried powering the system off then on?"
},
{
"repliedOn" : "2020-02-03T08:19:27-06:00",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "johnsmith@testco.com",
"message" : "Yes, I have."
}
]
}
Represents a ticket data object.
Fields
Field | Type | Description |
---|---|---|
ticketNumber | Integer | The reference number of the ticket. |
priority | String | The priority of the ticket. See Ticket Priorities for all possible values. |
status | String | The status of the ticket. See Ticket Statuses for all possible values |
firstName | String | The first name of the person creating the ticket |
lastName | String | The last name of the person creating the ticket |
emailAddress | String | The email address of the contact |
phoneNumber | String | The phone number of the person creating the ticket |
subject | String | The subject of the ticket |
message | String | The message body of the ticket |
replies[] | Array | An array of Ticket Reply objects. |
replies[]{}.repliedOn | Date/Time | The date/time when the ticket reply was made. (Defaults to "now" when creating.) |
replies[]{}.firstName | String | The first name of the person replying to the ticket |
replies[]{}.lastName | String | The last name of the person replying to the ticket |
replies[]{}.emailAddress | String | The email address of the person replying to the ticket |
replies[]{}.message | String | The message body of the ticket reply |
Ticket Reply
Sample Ticket Reply Object
{
"repliedOn" : "2020-02-03T07:34:16-06:00",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "janedoe@testco.com",
"message" : "Have you tried powering the system off then on?"
}
Represents a ticket reply data object.
Fields
Field | Type | Description |
---|---|---|
repliedOn | Date/Time | The date/time when the ticket reply was made. (Defaults to "now" when creating.) |
firstName | String | The first name of the person replying to the ticket |
lastName | String | The last name of the person replying to the ticket |
emailAddress | String | The email address of the person replying to the ticket |
message | String | The message body of the ticket reply |
Tickets
Create a TicketPOST /ticket
Use this request to create a ticket.
- This method expects one Ticket object to be passed in the request payload.
Sample Request
POST /ticket
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"priority" : "NORMAL",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "johnsmith@testco.com",
"phoneNumber" : "(123) 456-7890",
"subject" : "My computer won't boot up",
"message" : "I cannot get the computer to boot into Windows"
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
priority | String | The priority of the ticket. See Ticket Priorities for all possible values. | No |
firstName | String | The first name of the person creating the ticket | No |
lastName | String | The last name of the person creating the ticket | No |
emailAddress | String | The email address of the contact | Yes |
phoneNumber | String | The phone number of the person creating the ticket | No |
subject | String | The subject of the ticket | Yes |
message | String | The message body of the ticket | Yes |
Sample Response
HTTP/1.1 201 CREATED
{
"success" : true,
"error" : "",
"ticketNumber" : 12345678
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
ticketNumber | Integer | The ticket number which was created for referencing later |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Add a Ticket ReplyPOST /ticket/{ticketNumber}/reply
Use this request to add a reply messages to a ticket.
- The
{ticketNumber}
value in the route is an integer which references a previously created ticket number. - This method expects one Ticket Reply object to be passed in the request payload.
Sample Request
POST /ticket/12345678/reply
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"repliedOn" : "2020-02-03T07:34:16-06:00",
"emailAddress" : "janedoe@testco.com",
"firstName" : "Jane",
"lastName" : "Doe",
"message" : "Have you tried powering the system off then on?"
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
repliedOn | Date/Time | The date/time when the ticket reply was made. (Defaults to "now" when creating.) | No |
firstName | String | The first name of the person replying to the ticket | No |
lastName | String | The last name of the person replying to the ticket | No |
emailAddress | String | The email address of the person replying to the ticket | Yes |
message | String | The message body of the ticket reply | Yes |
Sample Response
HTTP/1.1 201 CREATED
{
"success" : true,
"error" : "",
"ticketNumber" : 12345678
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
ticketNumber | Integer | The ticket number of the record which the reply was posted to |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Update a TicketPUT /ticket/{ticketNumber}
Use this request to modify a ticket or update its status.
- The
{ticketNumber}
value in the route is an integer which references the prior ticket number created. - This method expects one Ticket object to be passed in the request payload.
- Only the fields passed will be modified. Any fields not passed will leave the value for that field unchanged.
Sample Request
PUT /ticket/12345678
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"priority" : "NORMAL",
"status" : "IN_PROGRESS",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "janedoe@testco.com",
"phoneNumber" : "(123) 456-7890",
"subject" : "My computer won't boot up",
"message" : "I cannot get the computer to boot into Windows"
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
priority | String | The priority of the ticket. See Ticket Priorities for all possible values. | No |
status | String | The status of the ticket. See Ticket Statuses for all possible values | No |
firstName | String | The first name of the person creating the ticket | No |
lastName | String | The last name of the person creating the ticket | No |
emailAddress | String | The email address of the contact | No |
phoneNumber | String | The phone number of the person creating the ticket | No |
subject | String | The subject of the ticket | No |
message | String | The message body of the ticket | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"ticketNumber" : 12345678
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
ticketNumber | Integer | The ticket number which was updated |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Retrieve a TicketGET /ticket/{ticketNumber}
Use this request to retrieve a ticket and view its status or information
- The
{ticketNumber}
value in the route is an integer which references the prior ticket number created.
Sample Request
GET /ticket/12345678
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"ticket" : {
"ticketNumber" : 12345678,
"priority" : "NORMAL",
"status" : "IN_PROGRESS",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "johnsmith@testco.com",
"phoneNumber" : "(123) 456-7890",
"subject" : "My computer won't boot up",
"message" : "I cannot get the computer to boot into Windows",
"replies" : [
{
"repliedOn" : "2020-02-03T07:34:16-06:00",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "janedoe@testco.com",
"message" : "Have you tried powering the system off then on?"
},
{
"repliedOn" : "2020-02-03T08:19:27-06:00",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "johnsmith@testco.com",
"message" : "Yes, I have."
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
ticket | Ticket | Contains the returned ticket details. |
ticket{}.ticketNumber | Integer | The reference number of the ticket. |
ticket{}.priority | String | The priority of the ticket. See Ticket Priorities for all possible values. |
ticket{}.status | String | The status of the ticket. See Ticket Statuses for all possible values |
ticket{}.firstName | String | The first name of the person creating the ticket |
ticket{}.lastName | String | The last name of the person creating the ticket |
ticket{}.emailAddress | String | The email address of the contact |
ticket{}.phoneNumber | String | The phone number of the person creating the ticket |
ticket{}.subject | String | The subject of the ticket |
ticket{}.message | String | The message body of the ticket |
ticket{}.replies[] | Array | An array of Ticket Reply objects. |
ticket{}.replies[]{}.repliedOn | Date/Time | The date/time when the ticket reply was made. (Defaults to "now" when creating.) |
ticket{}.replies[]{}.firstName | String | The first name of the person replying to the ticket |
ticket{}.replies[]{}.lastName | String | The last name of the person replying to the ticket |
ticket{}.replies[]{}.emailAddress | String | The email address of the person replying to the ticket |
ticket{}.replies[]{}.message | String | The message body of the ticket reply |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Webhooks
If a webhook has been configured with your account, Agilant Solutions will automatically POST
a JSON request to your provided endpoint when an event occurs.
- If specific authentication requirements are needed, please let Agilant Solutions know and the security requirements can likely be accommodated.
- Note that your server responses will not be processed by Agilant Solutions. Therefore, there is no retry policy for webhooks if your server responds unsuccessfully.
Ticket Status ChangePOST {YourEndpoint}
This webhook will trigger when the status of a ticket has changed.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "TICKET_STATUS_CHANGE",
"ticketNumber" : 12345678,
"status" : "CLOSED"
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly TICKET_STATUS_CHANGE . See Webhook Types for all possible webhook values. |
ticketNumber | Integer | The ticket number of the ticket that changed |
status | String | The new status of the ticket. See Ticket Statuses for all possible values |
Ticket ReplyPOST {YourEndpoint}
This webhook will trigger when a reply has been added to the ticket.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "TICKET_REPLY",
"ticketNumber" : 12345678,
"reply" : {
"repliedOn" : "2020-02-03T07:34:16-06:00",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "janedoe@testco.com",
"message" : "Have you tried restarting your machine?"
}
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly TICKET_REPLY . See Webhook Types for all possible webhook values. |
ticketNumber | Integer | The ticket number of the ticket that changed |
reply | Ticket Reply | The reply object that was added to the ticket. |
reply{}.repliedOn | Date/Time | The date/time when the ticket reply was made. (Defaults to "now" when creating.) |
reply{}.firstName | String | The first name of the person replying to the ticket |
reply{}.lastName | String | The last name of the person replying to the ticket |
reply{}.emailAddress | String | The email address of the person replying to the ticket |
reply{}.message | String | The message body of the ticket reply |