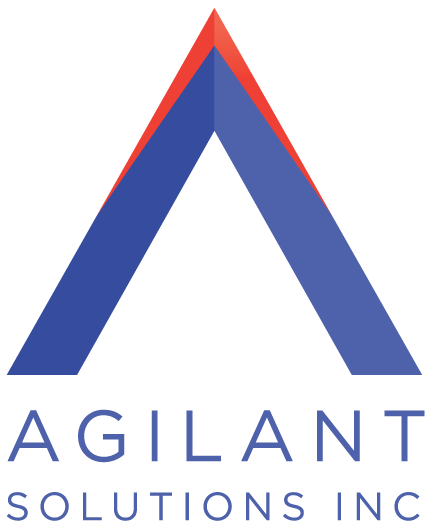
Overview
Introduction
This API implements functionality for utilizing the core features of Agilant’s TOGa system through a RESTful HTTP transaction containing a JSON payload.
Endpoints
Beta/Test/Sandbox Environmenthttps://api.beta.agilantsolutions.com
Production Environmenthttps://api.agilantsolutions.com
Structure & Schema
- All requests and responses are formatted in JSON notation.
- Any whitespace in the request payload is disregarded. The JSON examples in this document are shown in a "pretty print" structure for easier legibility but JSON is not required to be in "pretty print" when performing the actual transactions.
- This API is intended for businesses-to-businesses (B2B) and must be implemented on your server-side. Requests to this API should never be sent from or processed by a user on the client-side.
- There are no data or structural differences between our beta and production environment. The only change you must make to initiate a request in production is that it must be sent to our production URL endpoint.
- All parameter names are case-sensitive and all values are handled with case sensitivity.
- Connection timeouts should be set to 15 seconds.
- Date and times in any inputs and outputs are formatted in ISO 8601 notation.
- For example:
1994-11-05
corresponds to November 5, 1994. - For example:
1994-11-05T08:15:30-05:00
corresponds to November 5, 1994, 8:15:30 am, US Eastern Standard Time. - For example:
08:15:30-05:00
corresponds to 8:15:30 am, US Eastern Standard Time.
Authentication & Authorization
- During the onboarding process, Agilant will provide you with two GUID keys which you are to store. One key is a public API key and the other key is a secret API key. You must ensure that the secret key is not disclosed anywhere in your code to the public.
- In the header of every transaction, you will need to provide a timestamp, your public key, and a signature of your transaction comprising of the timestamp field signed by your secret API key using the HMAC-SHA-256 cryptographic hash algorithm.
- The timestamp must be within 120 seconds (2 minutes) of the actual time or your transaction will be rejected. Therefore, you must ensure that your system time is maintained accurately within 2 minutes of the actual universal time.
Required Request Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Must be set to application/json |
Timestamp | String | The current timestamp of your transaction in ISO 8601 notation. Example: 2019-01-02T17:34:52-05:00 |
Authorization | String | Concatenated string of your public API key, followed by a literal period (. ), followed by your signature. Your signature is your timestamp value, signed using your secret API key through the HMAC-SHA-256 cryptographic hash algorithm in a raw binary format and then Base64 encoded.Format: {publicKey}.{signatureOfTimestampUsingSecretKey} |
Sample Authentication Request Headers
Content-Type: application/json
Timestamp: 2019-01-02T17:34:52-05:00
Authorization: 1111AAAA-22BB-33CC-44DD-555555EEEEEE.Omjruf/UNd+rKEbjobxJzky84h6XOE9o9jz6/IKqc7Q=
Sample Authentication Request Headers
See the sample request using the sample information below.
- Using Sample Timestamp of:
2019-01-02T17:34:52-05:00
- Using Sample Public API Key of:
1111AAAA-22BB-33CC-44DD-555555EEEEEE
- Using Sample Secret API Key of:
EEEE5555-DD44-CC33-BB22-AAAAAA111111
Sample PHP Code for Assembling Request Headers
<?php
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
?>
Responses
- All responses include two fields called
success
anderror
. - The
success
field is a boolean data type which will returntrue
orfalse
for whether or not the transaction was successful. - The
error
field will contain an intelligible string of the error details if one occurred or will be an empty value if no error occurred. - Additionally, all transactions will respond with the following codes if they are successful:
200 OK
for allGET
,PUT
, andDELETE
requests.201 CREATED
for allPOST
requests.- When determining for whether or not the transaction was successful, you should check that the HTTP response code is
200 OK
or201 CREATED
and/or check that thesuccess
value is set totrue
.
Sample Response Headers
Content-Type: application/json
Content-Length: 354
Response Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Will always be set to application/json |
Content-Length | Integer | Length of the response payload in bytes. |
Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
401 UNAUTHORIZED | Authentication or authorization failed. | Review the returned errors and correct the issue. If the problem persists, you may not be permitted to perform this API call. |
403 FORBIDDEN | Access was denied when trying to retrieve data for a record. | Ensure you have proper authorization to access the record. |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
405 METHOD NOT ALLOWED | The requested action is not supported. | Ensure that you are calling a valid API method and using the correct method and route. |
408 REQUEST TIMEOUT | A connection error was encountered and the request may or may not have completed successfully. | Retry the transaction and/or perform other GET requests to check if the previous request succeeded. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
500 INTERNAL SERVER ERRROR | An unexpected internal server error was encountered. | Contact the administrator. Further analysis and debugging may need to be performed. |
502 BAD GATEWAY | A temporary network issue occurred when receiving the request. This is rare and typically caused by a very temporary network issue which should resolve within a few seconds. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
504 GATEWAY TIMEOUT | A network issue occurred when receiving the request. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
PHP Example
<?php
$endpoint = 'https://api.beta.agilantsolutions.com'; // SANDBOX ENDPOINT
//$endpoint = 'https://api.agilantsolutions.com'; // PRODUCTION ENDPOINT
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$method = 'GET';
$route = '/provider';
$payload = '{"providers":["C638C170-3EC1-4DF2-942C-1D1C3AD74FBC","16527A9E-4048-452CA8DF-605E1038A313"]}';
//////////////////////////////////////////////////
$url = ($endpoint . $route);
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, $method);
curl_setopt($ch, CURLOPT_POSTFIELDS, $payload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$responseBody = curl_exec($ch);
$responseCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
echo 'responseCode=' . $responseCode . '<br>';
echo 'responseBody=' . $responseBody;
///////////////////////////////////////////////
/*
EXAMPLE OUTPUT:
responseCode=200
responseBody=
{
"success": true,
"error": "",
"providers": [
{
"providerId": "C638C170-3EC1-4DF2-942C-1D1C3AD74FBC",
"providerName": "Porch",
"avgRating": 4.3,
"reviews": [
{
"name": "Jane Doe",
"rating": 4.7,
"review": "I like this service very much!"
},
{
"name": "John Smith",
"rating": 3.5,
"review": "I was quite happy!"
}
]
},
{
"providerId": "16527A9E-4048-452C-A8DF-605E1038A313",
"providerName": "Hello Tech",
"avgRating": 3.1,
"reviews": [
{
"name": "Mary Doe",
"rating": 2.7,
"review": "I was not happy with this service.
},
{
"name": "Jim Dean",
"rating": 4.1,
"review": "This looks really nice!"
}
]
}
]
}
*/
Enumerated Lists
Cart Statuses
Defines the overall status of the appointments within a cart.
Values
Value | Description |
---|---|
PENDING | The appointment window request is pending submission for processing |
IN_PROGRESS | The appointment window request is processing |
CONFIRMED | The appointment window request is confirmed |
COMPLETE | The appointment is completed |
CANCELLED | The appointment window request was cancelled |
Question Types
Defines the types of question.
Values
Value | Description |
---|---|
BOOLEAN | Should be shown as a YES/NO question |
RADIO | Should be shown as a radio question with multiple options where only one answer can be selected |
CHECKBOX | Should be shown as a checkbox question with multiple options where multiple answers can be selected |
DROPDOWN | Should be shown as a dropdown/select question with multiple options where onlt one answer can be selected |
TEXTFIELD | Should be shown as a single line text field allowing for manual user input |
TEXTAREA | Should be shown as a multiple line text area field allowing for manual user input |
Data Objects
Customer
Sample Customer Object
{
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
}
Represents a customer record.
Field | Type | Description |
---|---|---|
id | String | Your unique identifer of the customer record in your system to reference later. |
businessName | String | Customer's business name |
firstName | String | Customer's first name |
lastName | String | Customer's last name |
emailAddress | String | Customer's email address |
phoneNumber | String | Customer's phone number |
address1 | String | Customer's appointment service address line 1 |
address2 | String | Customer's appointment service address line 2 |
city | String | Customer's appointment city |
state | String | Customer's appointment state |
zipCode | String | Customer's appointment zipCode |
notes | String | Customer notes which will be passed through to the provider for the appointment |
Geolocation
Sample Geolocation Object
{
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
}
Represents a geographical location object.
Field | Type | Description |
---|---|---|
zipCode | String | ZIP code used for finding available services |
latitude | Float | Latitude to use for finding available services |
longitude | Float | Longitude to use for finding available services |
accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
Product
Sample Product Object
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
Represents a product object.
Field | Type | Description |
---|---|---|
id | String | Your unique product reference number or identifier to the product record in your system. |
departmentCode | String | Product department code |
classCode | String | Product class code |
subclassCode | String | Product subclass code |
familyCode | String | Product family code |
skuNumber | String | Product SKU number |
upcCode | String | Product UPC code |
manufacturer | String | Product manufacturer |
model | String | Product model number |
shortDescription | String | Short description of the product |
longDescription | String | Long description of the product |
scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
Availability
Sample Availability Object
{
"id" : "3e80732c-9c38-e5a8-fb0a-068b1404a1b8",
"serviceId" : "5df9fc2a-759e-8c2d-94e1-8ec82034db1a",
"providerId" : "e774d149-3170-2d59-a29e-1569a58b6525",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
}
Represents a service appointment availability object.
Field | Type | Description |
---|---|---|
id | String | Unique identifer of the availabilty record |
serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
providerId | String | Unique identifer of the provider record handling this appointment |
windowStart | Date/Time | Starting date/time of the appointment window |
windowEnd | Date/Time | Ending date/time of the appointment window |
Answer
Sample Answer Object
{
"id" : "e897c60c-8eee-c10f-f21c-e9b60499ffc1",
"answer" : "I have not backed up my system recently"
}
Represents an answer to a question object.
Field | Type | Description |
---|---|---|
id | String | Unique identifer of the answer record for a particular question |
answer | String | The answer option to be presented to the customer |
Question
Sample Question Object
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
}
Represents a product question object.
Field | Type | Description |
---|---|---|
id | String | Unique identifer of the question record |
productId | String | Your unique product reference number or identifier to the product record in your system. |
serviceId | String | Unique service reference identifier. |
visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
sortOrder | Integer | Sort order the question should appear in |
required | Boolean | Whether or not the question is required to be answered |
type | String | The type of question. See Question Types for all possible values. |
question | String | The question to be asked of the customer |
answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
answer[]{}.id | String | Unique identifer of the answer record for a particular question |
answer[]{}.answer | String | The answer option to be presented to the customer |
User Review
Sample Review Object
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
}
Represents a user review object.
Field | Type | Description |
---|---|---|
id | String | Unique identifier of the review record. |
name | String | Name of the reviewer |
rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
review | String | Text block of the user's review |
Provider
Sample Provider Object
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "c62e34fa-14bf-b11f-18d6-62bf9ff39ee1",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
}
Represents a provider object.
Field | Type | Description |
---|---|---|
id | String | Unique identifier of the provider record |
productId | String | Your identifier of the product this service is for. |
name | String | Provider's name |
cost | Float | Used within service records, this represents the unit cost of the service for that provider |
avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
review[]{}.id | String | Unique identifier of the review record. |
review[]{}.name | String | Name of the reviewer |
review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
review[]{}.review | String | Text block of the user's review |
Service
Sample Service Object
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
}
Represents a service object.
Field | Type | Description |
---|---|---|
id | String | Unique identifier of the service record |
productId | String | Your identifier of the product this service is for. |
preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
sortOrder | Integer | Sort order the service should appear in |
skuNumber | String | SKU number of the service |
upcCode | String | UPC code of the service |
price | Float | Unit sell price of the service |
shortDescription | String | Short description of the service |
longDescription | String | Long description of the service |
minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
provider[] | Array | An array of Provider objects capable of providing this service |
provider[]{}.id | String | Unique identifier of the provider record |
provider[]{}.productId | String | Your identifier of the product this service is for. |
provider[]{}.name | String | Provider's name |
provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
provider[]{}.review[]{}.name | String | Name of the reviewer |
provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[]{}.review | String | Text block of the user's review |
availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
availability[]{}.id | String | Unique identifer of the availabilty record |
availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Cart
Sample Cart Object
{
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
],
"answer" : [
"8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"ee6848ba-31d2-c827-eab3-ef1cadc2a624"
]
}
Represents a cart object.
Field | Type | Description |
---|---|---|
id | String | Your unique identifier of the cart record in your system to reference again later. |
status | String | Overall appointment status. See Cart Statuses for all possible values. |
purchaseOrder | String | Your purchase order number to pass through to the provider. |
customer{} | Customer | The customer object containing details this cart is for. |
customer{}.id | String | Your unique identifer of the customer record in your system to reference later. |
customer{}.businessName | String | Customer's business name |
customer{}.firstName | String | Customer's first name |
customer{}.lastName | String | Customer's last name |
customer{}.emailAddress | String | Customer's email address |
customer{}.phoneNumber | String | Customer's phone number |
customer{}.address1 | String | Customer's appointment service address line 1 |
customer{}.address2 | String | Customer's appointment service address line 2 |
customer{}.city | String | Customer's appointment city |
customer{}.state | String | Customer's appointment state |
customer{}.zipCode | String | Customer's appointment zipCode |
customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment |
geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. |
geolocation{}.zipCode | String | ZIP code used for finding available services |
geolocation{}.latitude | Float | Latitude to use for finding available services |
geolocation{}.longitude | Float | Longitude to use for finding available services |
geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
product[] | Array | An array of Product objects in the cart |
product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
product[]{}.departmentCode | String | Product department code |
product[]{}.classCode | String | Product class code |
product[]{}.subclassCode | String | Product subclass code |
product[]{}.familyCode | String | Product family code |
product[]{}.skuNumber | String | Product SKU number |
product[]{}.upcCode | String | Product UPC code |
product[]{}.manufacturer | String | Product manufacturer |
product[]{}.model | String | Product model number |
product[]{}.shortDescription | String | Short description of the product |
product[]{}.longDescription | String | Long description of the product |
product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
service[] | Array | An array of Service objects in the cart |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
question[] | Array | An array of Question objects representing the questions to be asked of the customer for the products in the cart. |
question[]{}.id | String | Unique identifer of the question record |
question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question[]{}.serviceId | String | Unique service reference identifier. |
question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question[]{}.sortOrder | Integer | Sort order the question should appear in |
question[]{}.required | Boolean | Whether or not the question is required to be answered |
question[]{}.type | String | The type of question. See Question Types for all possible values. |
question[]{}.question | String | The question to be asked of the customer |
question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. |
Products
Retrieve Product ServicesGET /product/services
Use this request to retrieve all services applicable to a product.
- This method expects one Product object to be passed in the request payload.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/services
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | Product to be used in lookup. | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Available ServicesGET /product/services/available
Use this request to retrieve all available services applicable to a product in a certain geographical location.
- This method expects a Product object and a Geolocation object to be passed in the request payload.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
- At least one geolocation input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/services/available
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
geolocation{} | Geolocation | The geolocation parameters used to find services around | Yes |
geolocation{}.zipCode | String | ZIP code used for finding available services | No |
geolocation{}.latitude | Float | Latitude to use for finding available services | No |
geolocation{}.longitude | Float | Longitude to use for finding available services | No |
geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"provider" : {
"0" : {
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
"1" : {
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
},
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
]
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Product ProvidersGET /product/providers
Use this request to retrieve all service provider details based upon a product.
- This method expects a Product object and a Geolocation object to be passed in the request payload.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
- At least one geolocation input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/providers
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
geolocation{} | Geolocation | The geolocation parameters used to find services around | Yes |
geolocation{}.zipCode | String | ZIP code used for finding available services | No |
geolocation{}.latitude | Float | Latitude to use for finding available services | No |
geolocation{}.longitude | Float | Longitude to use for finding available services | No |
geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
provider[] | Array | An array of Provider objects. |
provider[]{}.id | String | Unique identifier of the provider record |
provider[]{}.productId | String | Your identifier of the product this service is for. |
provider[]{}.name | String | Provider's name |
provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
provider[]{}.review[]{}.name | String | Name of the reviewer |
provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve RecommendationsGET /product/recommended
Use this request to retrieve both recommended services and products of a product.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/recommended
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
],
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)"
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)"
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of recommended Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
product[] | Array | An array of recommended Product objects. |
product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
product[]{}.departmentCode | String | Product department code |
product[]{}.classCode | String | Product class code |
product[]{}.subclassCode | String | Product subclass code |
product[]{}.familyCode | String | Product family code |
product[]{}.skuNumber | String | Product SKU number |
product[]{}.upcCode | String | Product UPC code |
product[]{}.manufacturer | String | Product manufacturer |
product[]{}.model | String | Product model number |
product[]{}.shortDescription | String | Short description of the product |
product[]{}.longDescription | String | Long description of the product |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Recommended ServicesGET /product/recommended/services
Use this request to retrieve recommended services for a product.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/recommended/services
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of recommended Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Recommended ProductsGET /product/recommended/products
Use this request to retrieve recommended products for a product.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/recommended/products
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
product{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)"
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)"
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
product[] | Array | An array of recommended Product objects. |
product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
product[]{}.departmentCode | String | Product department code |
product[]{}.classCode | String | Product class code |
product[]{}.subclassCode | String | Product subclass code |
product[]{}.familyCode | String | Product family code |
product[]{}.skuNumber | String | Product SKU number |
product[]{}.upcCode | String | Product UPC code |
product[]{}.manufacturer | String | Product manufacturer |
product[]{}.model | String | Product model number |
product[]{}.shortDescription | String | Short description of the product |
product[]{}.longDescription | String | Long description of the product |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Product QuestionsGET /product/questions
Use this request to retrieve questions to be answered by the customer for a product.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /product/questions
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"product" : {
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
product{} | Product | The product to use for locating available services | Yes |
product{}.id | String | Your unique product reference number or identifier to the product record in your system. | Yes |
product{}.departmentCode | String | Product department code | No |
product{}.classCode | String | Product class code | No |
product{}.subclassCode | String | Product subclass code | No |
product{}.familyCode | String | Product family code | No |
product{}.skuNumber | String | Product SKU number | No |
product{}.upcCode | String | Product UPC code | No |
product{}.manufacturer | String | Product manufacturer | No |
product{}.model | String | Product model number | No |
product{}.shortDescription | String | Short description of the product | No |
product{}.longDescription | String | Long description of the product | No |
product{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
question[] | Array | An array of Question objects. |
question[]{}.id | String | Unique identifer of the question record |
question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question[]{}.serviceId | String | Unique service reference identifier. |
question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question[]{}.sortOrder | Integer | Sort order the question should appear in |
question[]{}.required | Boolean | Whether or not the question is required to be answered |
question[]{}.type | String | The type of question. See Question Types for all possible values. |
question[]{}.question | String | The question to be asked of the customer |
question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Cart
Create a CartPOST /cart/{cart.id}
Use this request to save an initial cart and/or create a new appointment reservation.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
POST /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
],
"service" : [
"7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"d4c624b3-dc3b-e736-7590-721bb898480f"
],
"availability" : [
"54f42f62-2cff-5149-446b-60d6f2cb2b6d"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
cart{} | Cart | The cart data you are passing with this transaction. | Yes |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. | Yes |
cart{}.purchaseOrder | String | Your purchase order number to pass through to the provider. | No |
cart{}.customer{} | Customer | The customer object containing details this cart is for. | Yes |
cart{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. | Yes |
cart{}.customer{}.businessName | String | Customer's business name | No |
cart{}.customer{}.firstName | String | Customer's first name | No |
cart{}.customer{}.lastName | String | Customer's last name | No |
cart{}.customer{}.emailAddress | String | Customer's email address | No |
cart{}.customer{}.phoneNumber | String | Customer's phone number | No |
cart{}.customer{}.address1 | String | Customer's appointment service address line 1 | No |
cart{}.customer{}.address2 | String | Customer's appointment service address line 2 | No |
cart{}.customer{}.city | String | Customer's appointment city | No |
cart{}.customer{}.state | String | Customer's appointment state | No |
cart{}.customer{}.zipCode | String | Customer's appointment zipCode | No |
cart{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment | No |
cart{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. | No |
cart{}.geolocation{}.zipCode | String | ZIP code used for finding available services | No |
cart{}.geolocation{}.latitude | Float | Latitude to use for finding available services | No |
cart{}.geolocation{}.longitude | Float | Longitude to use for finding available services | No |
cart{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services | No |
cart{}.product[] | Array | An array of Product objects in the cart | No |
cart{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. | No |
cart{}.product[]{}.departmentCode | String | Product department code | No |
cart{}.product[]{}.classCode | String | Product class code | No |
cart{}.product[]{}.subclassCode | String | Product subclass code | No |
cart{}.product[]{}.familyCode | String | Product family code | No |
cart{}.product[]{}.skuNumber | String | Product SKU number | No |
cart{}.product[]{}.upcCode | String | Product UPC code | No |
cart{}.product[]{}.manufacturer | String | Product manufacturer | No |
cart{}.product[]{}.model | String | Product model number | No |
cart{}.product[]{}.shortDescription | String | Short description of the product | No |
cart{}.product[]{}.longDescription | String | Long description of the product | No |
cart{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
cart{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
cart{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. | No |
cart{}.service[] | Array | A simple array of service{}.id strings referencing the services in the cart. | |
cart{}.availability[] | Array | A simple array of availability{}.id strings referencing the availability windows chosen for the cart. |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
],
"answer" : [
"8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"ee6848ba-31d2-c827-eab3-ef1cadc2a624"
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart{} | Cart | Current snapshot of the cart object after your transaction. |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
cart{}.purchaseOrder | String | Your purchase order number to pass through to the provider. |
cart{}.customer{} | Customer | The customer object containing details this cart is for. |
cart{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. |
cart{}.customer{}.businessName | String | Customer's business name |
cart{}.customer{}.firstName | String | Customer's first name |
cart{}.customer{}.lastName | String | Customer's last name |
cart{}.customer{}.emailAddress | String | Customer's email address |
cart{}.customer{}.phoneNumber | String | Customer's phone number |
cart{}.customer{}.address1 | String | Customer's appointment service address line 1 |
cart{}.customer{}.address2 | String | Customer's appointment service address line 2 |
cart{}.customer{}.city | String | Customer's appointment city |
cart{}.customer{}.state | String | Customer's appointment state |
cart{}.customer{}.zipCode | String | Customer's appointment zipCode |
cart{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment |
cart{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. |
cart{}.geolocation{}.zipCode | String | ZIP code used for finding available services |
cart{}.geolocation{}.latitude | Float | Latitude to use for finding available services |
cart{}.geolocation{}.longitude | Float | Longitude to use for finding available services |
cart{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
cart{}.product[] | Array | An array of Product objects in the cart |
cart{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.product[]{}.departmentCode | String | Product department code |
cart{}.product[]{}.classCode | String | Product class code |
cart{}.product[]{}.subclassCode | String | Product subclass code |
cart{}.product[]{}.familyCode | String | Product family code |
cart{}.product[]{}.skuNumber | String | Product SKU number |
cart{}.product[]{}.upcCode | String | Product UPC code |
cart{}.product[]{}.manufacturer | String | Product manufacturer |
cart{}.product[]{}.model | String | Product model number |
cart{}.product[]{}.shortDescription | String | Short description of the product |
cart{}.product[]{}.longDescription | String | Long description of the product |
cart{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
cart{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
cart{}.service[] | Array | An array of Service objects in the cart |
cart{}.service[]{}.id | String | Unique identifier of the service record |
cart{}.service[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
cart{}.service[]{}.sortOrder | Integer | Sort order the service should appear in |
cart{}.service[]{}.skuNumber | String | SKU number of the service |
cart{}.service[]{}.upcCode | String | UPC code of the service |
cart{}.service[]{}.price | Float | Unit sell price of the service |
cart{}.service[]{}.shortDescription | String | Short description of the service |
cart{}.service[]{}.longDescription | String | Long description of the service |
cart{}.service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
cart{}.service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
cart{}.service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
cart{}.service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
cart{}.service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
cart{}.service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.provider[]{}.name | String | Provider's name |
cart{}.service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
cart{}.service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
cart{}.service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
cart{}.service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
cart{}.service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
cart{}.service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
cart{}.service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
cart{}.service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.question[] | Array | An array of Question objects representing the questions to be asked of the customer for the products in the cart. |
cart{}.question[]{}.id | String | Unique identifer of the question record |
cart{}.question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.question[]{}.serviceId | String | Unique service reference identifier. |
cart{}.question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
cart{}.question[]{}.sortOrder | Integer | Sort order the question should appear in |
cart{}.question[]{}.required | Boolean | Whether or not the question is required to be answered |
cart{}.question[]{}.type | String | The type of question. See Question Types for all possible values. |
cart{}.question[]{}.question | String | The question to be asked of the customer |
cart{}.question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
cart{}.question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
cart{}.question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
cart{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Change a CartPUT /cart/{cart.id}
Use this request to change a cart, or modify/cancel an appointment reservation of a cart{}.id
.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value. - Only the parameters passed will be modified.
Sample Request
PUT /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
],
"service" : [
"7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"d4c624b3-dc3b-e736-7590-721bb898480f"
],
"availability" : [
"54f42f62-2cff-5149-446b-60d6f2cb2b6d"
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
cart{} | Cart | The cart data you are passing with this transaction. | Yes |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. | No |
cart{}.purchaseOrder | String | Your purchase order number to pass through to the provider. | No |
cart{}.customer{} | Customer | The customer object containing details this cart is for. | No |
cart{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. | No |
cart{}.customer{}.businessName | String | Customer's business name | No |
cart{}.customer{}.firstName | String | Customer's first name | No |
cart{}.customer{}.lastName | String | Customer's last name | No |
cart{}.customer{}.emailAddress | String | Customer's email address | No |
cart{}.customer{}.phoneNumber | String | Customer's phone number | No |
cart{}.customer{}.address1 | String | Customer's appointment service address line 1 | No |
cart{}.customer{}.address2 | String | Customer's appointment service address line 2 | No |
cart{}.customer{}.city | String | Customer's appointment city | No |
cart{}.customer{}.state | String | Customer's appointment state | No |
cart{}.customer{}.zipCode | String | Customer's appointment zipCode | No |
cart{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment | No |
cart{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. | No |
cart{}.geolocation{}.zipCode | String | ZIP code used for finding available services | No |
cart{}.geolocation{}.latitude | Float | Latitude to use for finding available services | No |
cart{}.geolocation{}.longitude | Float | Longitude to use for finding available services | No |
cart{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services | No |
cart{}.product[] | Array | An array of Product objects in the cart | No |
cart{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. | No |
cart{}.product[]{}.departmentCode | String | Product department code | No |
cart{}.product[]{}.classCode | String | Product class code | No |
cart{}.product[]{}.subclassCode | String | Product subclass code | No |
cart{}.product[]{}.familyCode | String | Product family code | No |
cart{}.product[]{}.skuNumber | String | Product SKU number | No |
cart{}.product[]{}.upcCode | String | Product UPC code | No |
cart{}.product[]{}.manufacturer | String | Product manufacturer | No |
cart{}.product[]{}.model | String | Product model number | No |
cart{}.product[]{}.shortDescription | String | Short description of the product | No |
cart{}.product[]{}.longDescription | String | Long description of the product | No |
cart{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. | No |
cart{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. | No |
cart{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. | No |
cart{}.service[] | Array | A simple array of service{}.id strings referencing the services in the cart. | |
cart{}.availability[] | Array | A simple array of availability{}.id strings referencing the availability windows chosen for the cart. |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
],
"answer" : [
"8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"ee6848ba-31d2-c827-eab3-ef1cadc2a624"
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart{} | Cart | Current snapshot of the cart object after your transaction. |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
cart{}.purchaseOrder | String | Your purchase order number to pass through to the provider. |
cart{}.customer{} | Customer | The customer object containing details this cart is for. |
cart{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. |
cart{}.customer{}.businessName | String | Customer's business name |
cart{}.customer{}.firstName | String | Customer's first name |
cart{}.customer{}.lastName | String | Customer's last name |
cart{}.customer{}.emailAddress | String | Customer's email address |
cart{}.customer{}.phoneNumber | String | Customer's phone number |
cart{}.customer{}.address1 | String | Customer's appointment service address line 1 |
cart{}.customer{}.address2 | String | Customer's appointment service address line 2 |
cart{}.customer{}.city | String | Customer's appointment city |
cart{}.customer{}.state | String | Customer's appointment state |
cart{}.customer{}.zipCode | String | Customer's appointment zipCode |
cart{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment |
cart{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. |
cart{}.geolocation{}.zipCode | String | ZIP code used for finding available services |
cart{}.geolocation{}.latitude | Float | Latitude to use for finding available services |
cart{}.geolocation{}.longitude | Float | Longitude to use for finding available services |
cart{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
cart{}.product[] | Array | An array of Product objects in the cart |
cart{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.product[]{}.departmentCode | String | Product department code |
cart{}.product[]{}.classCode | String | Product class code |
cart{}.product[]{}.subclassCode | String | Product subclass code |
cart{}.product[]{}.familyCode | String | Product family code |
cart{}.product[]{}.skuNumber | String | Product SKU number |
cart{}.product[]{}.upcCode | String | Product UPC code |
cart{}.product[]{}.manufacturer | String | Product manufacturer |
cart{}.product[]{}.model | String | Product model number |
cart{}.product[]{}.shortDescription | String | Short description of the product |
cart{}.product[]{}.longDescription | String | Long description of the product |
cart{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
cart{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
cart{}.service[] | Array | An array of Service objects in the cart |
cart{}.service[]{}.id | String | Unique identifier of the service record |
cart{}.service[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
cart{}.service[]{}.sortOrder | Integer | Sort order the service should appear in |
cart{}.service[]{}.skuNumber | String | SKU number of the service |
cart{}.service[]{}.upcCode | String | UPC code of the service |
cart{}.service[]{}.price | Float | Unit sell price of the service |
cart{}.service[]{}.shortDescription | String | Short description of the service |
cart{}.service[]{}.longDescription | String | Long description of the service |
cart{}.service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
cart{}.service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
cart{}.service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
cart{}.service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
cart{}.service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
cart{}.service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.provider[]{}.name | String | Provider's name |
cart{}.service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
cart{}.service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
cart{}.service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
cart{}.service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
cart{}.service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
cart{}.service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
cart{}.service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
cart{}.service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.question[] | Array | An array of Question objects representing the questions to be asked of the customer for the products in the cart. |
cart{}.question[]{}.id | String | Unique identifer of the question record |
cart{}.question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.question[]{}.serviceId | String | Unique service reference identifier. |
cart{}.question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
cart{}.question[]{}.sortOrder | Integer | Sort order the question should appear in |
cart{}.question[]{}.required | Boolean | Whether or not the question is required to be answered |
cart{}.question[]{}.type | String | The type of question. See Question Types for all possible values. |
cart{}.question[]{}.question | String | The question to be asked of the customer |
cart{}.question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
cart{}.question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
cart{}.question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
cart{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Delete a CartDELETE /cart/{cart.id}
Use this request to delete a cart and cancel all appointment reservations of a cart{}.id
.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
DELETE /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : ""
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Retreive a CartGET /cart/{cart.id}
Use this request to retrieve cart and appointment details for a cart{}.id
.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
],
"answer" : [
"8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"ee6848ba-31d2-c827-eab3-ef1cadc2a624"
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart{} | Cart | Your requested cart details. |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
cart{}.purchaseOrder | String | Your purchase order number to pass through to the provider. |
cart{}.customer{} | Customer | The customer object containing details this cart is for. |
cart{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. |
cart{}.customer{}.businessName | String | Customer's business name |
cart{}.customer{}.firstName | String | Customer's first name |
cart{}.customer{}.lastName | String | Customer's last name |
cart{}.customer{}.emailAddress | String | Customer's email address |
cart{}.customer{}.phoneNumber | String | Customer's phone number |
cart{}.customer{}.address1 | String | Customer's appointment service address line 1 |
cart{}.customer{}.address2 | String | Customer's appointment service address line 2 |
cart{}.customer{}.city | String | Customer's appointment city |
cart{}.customer{}.state | String | Customer's appointment state |
cart{}.customer{}.zipCode | String | Customer's appointment zipCode |
cart{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment |
cart{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. |
cart{}.geolocation{}.zipCode | String | ZIP code used for finding available services |
cart{}.geolocation{}.latitude | Float | Latitude to use for finding available services |
cart{}.geolocation{}.longitude | Float | Longitude to use for finding available services |
cart{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
cart{}.product[] | Array | An array of Product objects in the cart |
cart{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.product[]{}.departmentCode | String | Product department code |
cart{}.product[]{}.classCode | String | Product class code |
cart{}.product[]{}.subclassCode | String | Product subclass code |
cart{}.product[]{}.familyCode | String | Product family code |
cart{}.product[]{}.skuNumber | String | Product SKU number |
cart{}.product[]{}.upcCode | String | Product UPC code |
cart{}.product[]{}.manufacturer | String | Product manufacturer |
cart{}.product[]{}.model | String | Product model number |
cart{}.product[]{}.shortDescription | String | Short description of the product |
cart{}.product[]{}.longDescription | String | Long description of the product |
cart{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
cart{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
cart{}.service[] | Array | An array of Service objects in the cart |
cart{}.service[]{}.id | String | Unique identifier of the service record |
cart{}.service[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
cart{}.service[]{}.sortOrder | Integer | Sort order the service should appear in |
cart{}.service[]{}.skuNumber | String | SKU number of the service |
cart{}.service[]{}.upcCode | String | UPC code of the service |
cart{}.service[]{}.price | Float | Unit sell price of the service |
cart{}.service[]{}.shortDescription | String | Short description of the service |
cart{}.service[]{}.longDescription | String | Long description of the service |
cart{}.service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
cart{}.service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
cart{}.service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
cart{}.service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
cart{}.service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
cart{}.service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
cart{}.service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
cart{}.service[]{}.provider[]{}.name | String | Provider's name |
cart{}.service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
cart{}.service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
cart{}.service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
cart{}.service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
cart{}.service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
cart{}.service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
cart{}.service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
cart{}.service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
cart{}.service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
cart{}.service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart{}.service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart{}.service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart{}.service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart{}.question[] | Array | An array of Question objects representing the questions to be asked of the customer for the products in the cart. |
cart{}.question[]{}.id | String | Unique identifer of the question record |
cart{}.question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
cart{}.question[]{}.serviceId | String | Unique service reference identifier. |
cart{}.question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
cart{}.question[]{}.sortOrder | Integer | Sort order the question should appear in |
cart{}.question[]{}.required | Boolean | Whether or not the question is required to be answered |
cart{}.question[]{}.type | String | The type of question. See Question Types for all possible values. |
cart{}.question[]{}.question | String | The question to be asked of the customer |
cart{}.question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
cart{}.question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
cart{}.question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
cart{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Retreive CartsGET /cart
Use this request to retrieve cart and appointment details for multiple cart{}.id
s.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
GET /cart
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"cart" : [
"e2b99b5e-6b64-d72f-c761-c87abe52f152"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
cart[] | Array | A simple array of cart{}.id strings to return cart details on. |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : [
{
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED",
"purchaseOrder" : "46273279ABC",
"customer" : {
"id" : "3b8cfce7-e219-e4f9-b1d6-c95c589380e4",
"businessName" : "XYZ Industries",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@xyzindustries.com",
"phoneNumber" : "123-456-7890",
"address1" : "123 Test Street",
"address2" : "Suite 543",
"city" : "Testville",
"state" : "TN",
"zipCode" : "92956",
"notes" : "Please call upon arrival"
},
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
},
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
],
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
],
"answer" : [
"8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"ee6848ba-31d2-c827-eab3-ef1cadc2a624"
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart[] | Array | An array of the cart objects requested. |
cart[]{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart[]{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
cart[]{}.purchaseOrder | String | Your purchase order number to pass through to the provider. |
cart[]{}.customer{} | Customer | The customer object containing details this cart is for. |
cart[]{}.customer{}.id | String | Your unique identifer of the customer record in your system to reference later. |
cart[]{}.customer{}.businessName | String | Customer's business name |
cart[]{}.customer{}.firstName | String | Customer's first name |
cart[]{}.customer{}.lastName | String | Customer's last name |
cart[]{}.customer{}.emailAddress | String | Customer's email address |
cart[]{}.customer{}.phoneNumber | String | Customer's phone number |
cart[]{}.customer{}.address1 | String | Customer's appointment service address line 1 |
cart[]{}.customer{}.address2 | String | Customer's appointment service address line 2 |
cart[]{}.customer{}.city | String | Customer's appointment city |
cart[]{}.customer{}.state | String | Customer's appointment state |
cart[]{}.customer{}.zipCode | String | Customer's appointment zipCode |
cart[]{}.customer{}.notes | String | Customer notes which will be passed through to the provider for the appointment |
cart[]{}.geolocation{} | Geolocation | The geolocation object containing location details of this cart which is used for finding availability. |
cart[]{}.geolocation{}.zipCode | String | ZIP code used for finding available services |
cart[]{}.geolocation{}.latitude | Float | Latitude to use for finding available services |
cart[]{}.geolocation{}.longitude | Float | Longitude to use for finding available services |
cart[]{}.geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services |
cart[]{}.product[] | Array | An array of Product objects in the cart |
cart[]{}.product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
cart[]{}.product[]{}.departmentCode | String | Product department code |
cart[]{}.product[]{}.classCode | String | Product class code |
cart[]{}.product[]{}.subclassCode | String | Product subclass code |
cart[]{}.product[]{}.familyCode | String | Product family code |
cart[]{}.product[]{}.skuNumber | String | Product SKU number |
cart[]{}.product[]{}.upcCode | String | Product UPC code |
cart[]{}.product[]{}.manufacturer | String | Product manufacturer |
cart[]{}.product[]{}.model | String | Product model number |
cart[]{}.product[]{}.shortDescription | String | Short description of the product |
cart[]{}.product[]{}.longDescription | String | Long description of the product |
cart[]{}.product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
cart[]{}.product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
cart[]{}.service[] | Array | An array of Service objects in the cart |
cart[]{}.service[]{}.id | String | Unique identifier of the service record |
cart[]{}.service[]{}.productId | String | Your identifier of the product this service is for. |
cart[]{}.service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
cart[]{}.service[]{}.sortOrder | Integer | Sort order the service should appear in |
cart[]{}.service[]{}.skuNumber | String | SKU number of the service |
cart[]{}.service[]{}.upcCode | String | UPC code of the service |
cart[]{}.service[]{}.price | Float | Unit sell price of the service |
cart[]{}.service[]{}.shortDescription | String | Short description of the service |
cart[]{}.service[]{}.longDescription | String | Long description of the service |
cart[]{}.service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
cart[]{}.service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
cart[]{}.service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
cart[]{}.service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
cart[]{}.service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
cart[]{}.service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
cart[]{}.service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
cart[]{}.service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
cart[]{}.service[]{}.provider[]{}.name | String | Provider's name |
cart[]{}.service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
cart[]{}.service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
cart[]{}.service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
cart[]{}.service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
cart[]{}.service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
cart[]{}.service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
cart[]{}.service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
cart[]{}.service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
cart[]{}.service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
cart[]{}.service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart[]{}.service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart[]{}.service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart[]{}.service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart[]{}.service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
cart[]{}.service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
cart[]{}.service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
cart[]{}.service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
cart[]{}.service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
cart[]{}.service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
cart[]{}.question[] | Array | An array of Question objects representing the questions to be asked of the customer for the products in the cart. |
cart[]{}.question[]{}.id | String | Unique identifer of the question record |
cart[]{}.question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
cart[]{}.question[]{}.serviceId | String | Unique service reference identifier. |
cart[]{}.question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
cart[]{}.question[]{}.sortOrder | Integer | Sort order the question should appear in |
cart[]{}.question[]{}.required | Boolean | Whether or not the question is required to be answered |
cart[]{}.question[]{}.type | String | The type of question. See Question Types for all possible values. |
cart[]{}.question[]{}.question | String | The question to be asked of the customer |
cart[]{}.question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
cart[]{}.question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
cart[]{}.question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
cart[]{}.answer[] | Array | A simple array of answer{}.id values which have been answered by the customer and are used to locate applicable products. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Retrieve Cart ServicesGET /cart/{cart.id}/services
Use this request to retrieve all applicable services to all products in the cart.
- This method expects Product objects to be passed in the
products
parameter in the request payload. - At least one product input parameter is required for each product but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/services
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Available ServicesGET /cart/{cart.id}/services/available
Use this request to retrieve all available services applicable to products in a cart in a certain geographical location.
- This method expects Product objects and a Geolocation object to be passed in the request payload.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
- At least one geolocation input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/services/available
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Cart ProvidersGET /cart/{cart.id}/providers
Use this request to retrieve all service provider details for products in a cart.
- This method expects Product objects and a Geolocation object to be passed in the request payload.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
- At least one geolocation input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/providers
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
provider[] | Array | An array of Provider objects. |
provider[]{}.id | String | Unique identifier of the provider record |
provider[]{}.productId | String | Your identifier of the product this service is for. |
provider[]{}.name | String | Provider's name |
provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
provider[]{}.review[]{}.name | String | Name of the reviewer |
provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve RecommendationsGET /cart/{cart.id}/recommended
Use this request to retrieve both recommended services and products of products in a cart.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/recommended
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
],
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of recommended Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
product[] | Array | An array of recommended Product objects. |
product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
product[]{}.departmentCode | String | Product department code |
product[]{}.classCode | String | Product class code |
product[]{}.subclassCode | String | Product subclass code |
product[]{}.familyCode | String | Product family code |
product[]{}.skuNumber | String | Product SKU number |
product[]{}.upcCode | String | Product UPC code |
product[]{}.manufacturer | String | Product manufacturer |
product[]{}.model | String | Product model number |
product[]{}.shortDescription | String | Short description of the product |
product[]{}.longDescription | String | Long description of the product |
product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Recommended ServicesGET /cart/{cart.id}/recommended/services
Use this request to retrieve recommended services for products in a cart.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/recommended/services
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of recommended Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Recommended ProductsGET /cart/{cart.id}/recommended/products
Use this request to retrieve recommended products for products in a cart.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/recommende/products
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"product" : [
{
"id" : "73316544-3729-c452-b3f3-83223529369e",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS140E-03CU8",
"upcCode" : "89072345907825967245",
"manufacturer" : "Toshiba",
"model" : "Satellite 1400-153E",
"shortDescription" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"scrubData" : "Toshiba Satellite 1400-153E, 1.33 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg) Toshiba Satellite 1400-153E. Processor frequency: 1.33 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 7.5 lbs (3.4 kg)",
"answer" : [
"71ee9886-48b9-6ffb-7fc4-c5008586c848",
"99e01d04-c154-e813-11b8-7ac70f4ee179"
]
},
{
"id" : "51b7e3a4-ca48-0d6e-5aaf-ac6286a71a59",
"departmentCode" : "200",
"classCode" : "210",
"subclassCode" : "220",
"familyCode" : "260",
"skuNumber" : "PS460E-079K9",
"upcCode" : "78569386973496791038",
"manufacturer" : "Toshiba",
"model" : "Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD",
"shortDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB",
"longDescription" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"scrubData" : "Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD, 0.75 GHz, 14.1", 1024 x 768 pixels, 20 GB Toshiba Satellite Pro 4600 PIII750/128/20/14.1TFT/DVD. Processor frequency: 0.75 GHz. Display diagonal: 14.1", Display resolution: 1024 x 768 pixels. Total storage capacity: 20 GB. Weight: 6.99 lbs (3.17 kg)",
"answer" : [
"1cb93869-d385-b79c-09a3-7e1bbd58d681",
"14d6ebe8-ca7b-42ce-a426-3b0286b57c50",
"c128b155-203b-da68-2cbf-0f7394ee5af0"
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
product[] | Array | An array of recommended Product objects. |
product[]{}.id | String | Your unique product reference number or identifier to the product record in your system. |
product[]{}.departmentCode | String | Product department code |
product[]{}.classCode | String | Product class code |
product[]{}.subclassCode | String | Product subclass code |
product[]{}.familyCode | String | Product family code |
product[]{}.skuNumber | String | Product SKU number |
product[]{}.upcCode | String | Product UPC code |
product[]{}.manufacturer | String | Product manufacturer |
product[]{}.model | String | Product model number |
product[]{}.shortDescription | String | Short description of the product |
product[]{}.longDescription | String | Long description of the product |
product[]{}.scrubData | String | Long text block of the product details used to scrub and locate applicable services. |
product[]{}.answer[] | Array | A simple array of answer{}.id strings which have been answered by the customer and is used to locate applicable services. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Cart QuestionsGET /cart/{cart.id}/questions
Use this request to retrieve questions to be answered by the customer for products in a cart.
- At least one product input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/questions
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
question[] | Array | An array of Question objects. |
question[]{}.id | String | Unique identifer of the question record |
question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question[]{}.serviceId | String | Unique service reference identifier. |
question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question[]{}.sortOrder | Integer | Sort order the question should appear in |
question[]{}.required | Boolean | Whether or not the question is required to be answered |
question[]{}.type | String | The type of question. See Question Types for all possible values. |
question[]{}.question | String | The question to be asked of the customer |
question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve AvailabilityGET /cart/{cart.id}/availability
Use this request to retrieve available appointment windows in a geolocation for products in a cart.
- At least one geolocation input parameter is required but providing more or all parameters will improve accuracy.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/availability
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
],
"availability" : [
{
"id" : "3a8cec43-e477-9137-6aa2-9e16cf4d6960",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-01T17:30:00-05:00"
},
{
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
},
{
"id" : "f43f65fd-7fa5-e50f-6a37-7e8e29560c86",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"windowStart" : "2020-07-01T15:30:00-05:00",
"windowEnd" : "2020-07-02T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "dbd7029f-b79a-55a4-08f8-24b03cc0ec56",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-01T17:30:00-05:00",
"windowEnd" : "2020-07-01T19:30:00-05:00"
}
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
],
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
],
"confirmedAvailability" : {
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects containing availability details. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.minAvailabilityWindows | Integer | The minimum number of availability windows the customer needs to select to create an appointment. |
service[]{}.maxAvailabilityWindows | Integer | The maximum number of availability windows the customer needs to select to create an appointment. |
service[]{}.nextAvailableProviderId | String | Used within calls that utilize a Geolocation object. Identifer of the provider with the next available appointment window. |
service[]{}.nextAvailableStart | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window start date/time. |
service[]{}.nextAvailableEnd | Date/Time | Used within calls that utilize a Geolocation object. Next available appointment window end date/time. |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
service[]{}.availability[] | Array | An array of Availability objects representing available appointment windows for this service. |
service[]{}.availability[]{}.id | String | Unique identifer of the availabilty record |
service[]{}.availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
service[]{}.confirmedAvailability{} | Availability | Availability object of the confirmed appointment |
service[]{}.confirmedAvailability{}.id | String | Unique identifer of the availabilty record |
service[]{}.confirmedAvailability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
service[]{}.confirmedAvailability{}.providerId | String | Unique identifer of the provider record handling this appointment |
service[]{}.confirmedAvailability{}.windowStart | Date/Time | Starting date/time of the appointment window |
service[]{}.confirmedAvailability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retreive Cart StatusesGET /cart/status
Use this request to retrieve only the cart status of multiple cart{}.id
strings.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
GET /cart/status
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"cart" : [
"e2b99b5e-6b64-d72f-c761-c87abe52f152",
"7f9d5988-d6fc-69eb-69f3-4469ca3b61a1"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
cart[] | Array | A simple array of cart{}.id strings to return cart details on. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : [
{
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED"
},
{
"id" : "7f9d5988-d6fc-69eb-69f3-4469ca3b61a1",
"status" : "COMPLETE"
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart[] | Array | An array of the cart objects requested. |
cart[]{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart[]{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Retreive a Cart's StatusGET /cart/{cart.id}/status
Use this request to retrieve only the cart status of one cart{}.id
.
- The
cart{}.id
field is a unique identifying string defined by you to reference the cart later. Typically, an order or transaction number is used for this value.
Sample Request
GET /cart/e2b99b5e-6b64-d72f-c761-c87abe52f152/status
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"cart" : {
"id" : "e2b99b5e-6b64-d72f-c761-c87abe52f152",
"status" : "CONFIRMED"
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
cart{} | Array | An array of the cart objects requested. |
cart{}.id | String | Your unique identifier of the cart record in your system to reference again later. |
cart{}.status | String | Overall appointment status. See Cart Statuses for all possible values. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Services
Retrieve ServicesGET /service
Use this request to retrieve the service details of multiple service{}.id
records.
Sample Request
GET /service
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"service" : [
"7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"d4c624b3-dc3b-e736-7590-721bb898480f"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
service[] | Array | A simply array of service{}.id values to retrieve details on. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : [
{
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
},
{
"id" : "d4c624b3-dc3b-e736-7590-721bb898480f",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 2,
"skuNumber" : "IHIO-SMARTTV",
"upcCode" : "234527259871235915",
"price" : 129.99,
"shortDescription" : "In-Home Smart TV Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 2,
"nextAvailableProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"nextAvailableStart" : "2020-10-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-10-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service[] | Array | An array of Service objects. |
service[]{}.id | String | Unique identifier of the service record |
service[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service[]{}.sortOrder | Integer | Sort order the service should appear in |
service[]{}.skuNumber | String | SKU number of the service |
service[]{}.upcCode | String | UPC code of the service |
service[]{}.price | Float | Unit sell price of the service |
service[]{}.shortDescription | String | Short description of the service |
service[]{}.longDescription | String | Long description of the service |
service[]{}.provider[] | Array | An array of Provider objects capable of providing this service |
service[]{}.provider[]{}.id | String | Unique identifier of the provider record |
service[]{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service[]{}.provider[]{}.name | String | Provider's name |
service[]{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service[]{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service[]{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service[]{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service[]{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service[]{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve a ServiceGET /service/{service.id}
Use this request to retrieve the service details of a service{}.id
record.
Sample Request
GET /service/7f038d35-81d2-8a30-ef00-4a30c8674d4a
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"service" : {
"id" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"preferredProviderId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"sortOrder" : 1,
"skuNumber" : "IHIO-DOORBELL",
"upcCode" : "823567917726452456",
"price" : 99.99,
"shortDescription" : "In-Home Smart Doorbell Installation",
"longDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"minAvailabilityWindows" : 1,
"maxAvailabilityWindows" : 3,
"nextAvailableProviderId" : "65d7299b-d4f7-9a57-c56b-ef1761e2851f",
"nextAvailableStart" : "2020-09-01T14:00:00-05:00",
"nextAvailableEnd" : "2020-09-01T16:00:00-05:00",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 49.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "d144e67d-2bce-dc3d-2c7c-d459469a1131",
"name" : "Jane Doe",
"rating" : 5,
"review" : "This service is great! I use it all the time!"
},
{
"id" : "2cf22bbd-9a49-f5b3-fb35-ac18969ff7fb",
"name" : "James Smith",
"rating" : 4,
"review" : "Very polite and knoweledgable technician! Thanks!"
}
]
},
{
"id" : "fc4b36ce-c561-fea8-7bde-6e216be58e54",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 39.99,
"avgRating" : 3.5,
"review" : [
{
"id" : "be96cb14-37c7-d44d-35e7-36149e9db49f",
"name" : "Debby Smith",
"rating" : 3,
"review" : "They were all right, I'd probably use them again."
},
{
"id" : "02c6a553-1144-94ac-012d-8f4b57253a8b",
"name" : "Todd Peterson",
"rating" : 4,
"review" : "Was quite happy with the way it turned out!"
}
]
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
service{} | Service | The service object containing your requested details. |
service{}.id | String | Unique identifier of the service record |
service{}.productId | String | Your identifier of the product this service is for. |
service{}.preferredProviderId | String | Identifier of the provider who is most preferred according to your business logic |
service{}.sortOrder | Integer | Sort order the service should appear in |
service{}.skuNumber | String | SKU number of the service |
service{}.upcCode | String | UPC code of the service |
service{}.price | Float | Unit sell price of the service |
service{}.shortDescription | String | Short description of the service |
service{}.longDescription | String | Long description of the service |
service{}.provider[] | Array | An array of Provider objects capable of providing this service |
service{}.provider[]{}.id | String | Unique identifier of the provider record |
service{}.provider[]{}.productId | String | Your identifier of the product this service is for. |
service{}.provider[]{}.name | String | Provider's name |
service{}.provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
service{}.provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
service{}.provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
service{}.provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
service{}.provider[]{}.review[]{}.name | String | Name of the reviewer |
service{}.provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
service{}.provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Service QuestionsGET /service/{service.id}/questions
Use this request to retrieve the questions to be answered by the customer for a service{}.id
record.
Sample Request
GET /service/7f038d35-81d2-8a30-ef00-4a30c8674d4a/questions
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
question[] | Array | An array of Question objects. |
question[]{}.id | String | Unique identifer of the question record |
question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question[]{}.serviceId | String | Unique service reference identifier. |
question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question[]{}.sortOrder | Integer | Sort order the question should appear in |
question[]{}.required | Boolean | Whether or not the question is required to be answered |
question[]{}.type | String | The type of question. See Question Types for all possible values. |
question[]{}.question | String | The question to be asked of the customer |
question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve Service AvailabilityGET /service/{service.id}/availability
Use this request to retrieve available appointment windows using geolocation information for a service{}.id
record.
Sample Request
GET /service/9b2f1470-c5da-2223-57a3-7c19928b57e6/availability
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"geolocation" : {
"zipCode" : "92956",
"latitude" : 53.36236,
"longitude" : -6.261633,
"accuracy" : 150
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
geolocation{} | Geolocation | The location of the customer used for finding availability. | Yes |
geolocation{}.zipCode | String | ZIP code used for finding available services | Yes |
geolocation{}.latitude | Float | Latitude to use for finding available services | No |
geolocation{}.longitude | Float | Longitude to use for finding available services | No |
geolocation{}.accuracy | Integer | Estimated accuracy (in meters) of radius around the latitude/longitude to use for finding available services | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
availability[] | Array | Array of Availability objects. |
availability[]{}.id | String | Unique identifer of the availabilty record |
availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Availability
Retrieve an AvailabilityGET /availability/{availability.id}
Use this request to retrieve appointment window availability details of one availability{}.id
string.
Sample Request
GET /availability/75e9c27f-e10f-4be9-b12a-fe838df53cfc
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"availability" : {
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
availability{} | Availability | Object contining the availability details. |
availability{}.id | String | Unique identifer of the availabilty record |
availability{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
availability{}.providerId | String | Unique identifer of the provider record handling this appointment |
availability{}.windowStart | Date/Time | Starting date/time of the appointment window |
availability{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve AvailabilitiesGET /availability
Use this request to retrieve appointment window availability details of multiple availability{}.id
strings.
Sample Request
GET /availability
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"availability" : [
"75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"54f42f62-2cff-5149-446b-60d6f2cb2b6d"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
availability[] | Array | A simply array of availability{}.id values to retrieve details on. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"availability" : [
{
"id" : "75e9c27f-e10f-4be9-b12a-fe838df53cfc",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-09T15:30:00-05:00",
"windowEnd" : "2020-07-09T17:30:00-05:00"
},
{
"id" : "b4a46ba1-24ab-c1ed-d6ad-15205a348426",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"windowStart" : "2020-07-09T17:30:00-05:00",
"windowEnd" : "2020-07-09T19:30:00-05:00"
},
{
"id" : "54f42f62-2cff-5149-446b-60d6f2cb2b6d",
"serviceId" : "7f038d35-81d2-8a30-ef00-4a30c8674d4a",
"providerId" : "7675bca8-0891-5667-3421-30fb64165aa3",
"windowStart" : "2020-07-10T15:30:00-05:00",
"windowEnd" : "2020-07-10T17:30:00-05:00"
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
availability[] | Array | An array of Availability objects representing the available appointment windows. |
availability[]{}.id | String | Unique identifer of the availabilty record |
availability[]{}.serviceId | String | Used within calls returning multiple service records. This is the unique identifier of the service this Availability record is for. |
availability[]{}.providerId | String | Unique identifer of the provider record handling this appointment |
availability[]{}.windowStart | Date/Time | Starting date/time of the appointment window |
availability[]{}.windowEnd | Date/Time | Ending date/time of the appointment window |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Providers
Retrieve a ProviderGET /provider/{provider.id}
Use this request to retrieve provider details of one provider{}.id
string.
Sample Request
GET /provider/7675bca8-0891-5667-3421-30fb64165aa3
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"provider" : {
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
provider{} | Provider | Object containing requested provider details. |
provider{}.id | String | Unique identifier of the provider record |
provider{}.productId | String | Your identifier of the product this service is for. |
provider{}.name | String | Provider's name |
provider{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
provider{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
provider{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
provider{}.review[]{}.id | String | Unique identifier of the review record. |
provider{}.review[]{}.name | String | Name of the reviewer |
provider{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
provider{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve ProvidersGET /provider
Use this request to retrieve provider details of multiple provider{}.id
strings.
Sample Request
GET /provider
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"provider" : [
"05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"7675bca8-0891-5667-3421-30fb64165aa3"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
provider[] | Array | A simply array of provider{}.id values to retrieve details on. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"provider" : [
{
"id" : "05cce2b2-1b0b-02f8-c01e-406bc1c7e60b",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Install Guys",
"cost" : 89.99,
"avgRating" : 4.5,
"review" : [
{
"id" : "45f21b40-66f9-d635-390b-71aa05cb8247",
"name" : "Sally Stewart",
"rating" : 5,
"review" : "Very professional job, thank you!"
},
{
"id" : "6941dab0-eda4-5bf1-3b9b-a03c3cfe729b",
"name" : "Donna Meadows",
"rating" : 4,
"review" : "Happy with my TV installation!"
}
]
},
{
"id" : "7675bca8-0891-5667-3421-30fb64165aa3",
"productId" : "b25e54a4-c244-e904-a5ac-2a0a16835885",
"name" : "Autohome Inc",
"cost" : 69.99,
"avgRating" : 3,
"review" : [
{
"id" : "1a127de1-e395-bc5b-cb79-b524545f5b96",
"name" : "Albert Macintosh",
"rating" : 3,
"review" : "Looks pretty good, they did scuff up my wall a little but otherwise, I'm happy."
},
{
"id" : "407c4d90-5963-d102-6b01-33f1c95c2661",
"name" : "Bob Fancy",
"rating" : 3,
"review" : "A few minutes late to show up but they did a nice job!"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
provider[] | Array | An array of Provider objects containing the provider details. |
provider[]{}.id | String | Unique identifier of the provider record |
provider[]{}.productId | String | Your identifier of the product this service is for. |
provider[]{}.name | String | Provider's name |
provider[]{}.cost | Float | Used within service records, this represents the unit cost of the service for that provider |
provider[]{}.avgRating | Float | Used within service records, this represents the average provider rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[] | Array | Used within service records, this is an array of the most recent provider reviews for this service |
provider[]{}.review[]{}.id | String | Unique identifier of the review record. |
provider[]{}.review[]{}.name | String | Name of the reviewer |
provider[]{}.review[]{}.rating | Float | User rating of the service on a scale between 1.0 and 5.0 |
provider[]{}.review[]{}.review | String | Text block of the user's review |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Questions
Retrieve a QuestionGET /question/{question.id}
Use this request to retrieve question details of one question{}.id
string.
Sample Request
GET /question/15e43784-fe92-51bf-6176-eb0f2abbf0b2
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"question" : {
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
question{} | Question | Object containing requested question details. |
question{}.id | String | Unique identifer of the question record |
question{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question{}.serviceId | String | Unique service reference identifier. |
question{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question{}.sortOrder | Integer | Sort order the question should appear in |
question{}.required | Boolean | Whether or not the question is required to be answered |
question{}.type | String | The type of question. See Question Types for all possible values. |
question{}.question | String | The question to be asked of the customer |
question{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question{}.answer[]{}.answer | String | The answer option to be presented to the customer |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
Retrieve QuestionsGET /question
Use this request to retrieve question details of multiple question{}.id
strings.
Sample Request
GET /question
Content-Type: application/json
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"question" : [
"15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"9b2f1470-c5da-2223-57a3-7c19928b57e6"
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
question[] | Array | A simply array of question{}.id values to retrieve details on. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : "",
"question" : [
{
"id" : "15e43784-fe92-51bf-6176-eb0f2abbf0b2",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 1,
"required" : true,
"type" : "BOOLEAN",
"question" : "Is the installation location on ground level?",
"answer" : [
{
"id" : "8d37ddf0-08e6-01cd-03f0-b3097bbad16a",
"answer" : "Yes"
},
{
"id" : "e18b9c0e-f2b3-d586-89e7-3f8bc276dc05",
"answer" : "No"
}
]
},
{
"id" : "9b2f1470-c5da-2223-57a3-7c19928b57e6",
"productId" : "9b5b728a-f578-1a6e-8942-a63a3ab2f5f8",
"serviceId" : "4ce443da-53ec-d878-1037-ee3542fadef9",
"visibleOnAnswerId" : null,
"sortOrder" : 2,
"required" : true,
"type" : "DROPDOWN",
"question" : "Approximately how heavy is the smart TV?",
"answer" : [
{
"id" : "573b972a-57b5-e17d-3645-a066e85b9b4a",
"answer" : "Under 25 pounds"
},
{
"id" : "ee6848ba-31d2-c827-eab3-ef1cadc2a624",
"answer" : "25-50 pounds"
},
{
"id" : "f7df97d0-9167-7ad2-58cb-15ed1aca3553",
"answer" : "Over 50 pounds"
}
]
}
]
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be empty if no error occurred. |
question[] | Array | An array of Question objects containing the question details. |
question[]{}.id | String | Unique identifer of the question record |
question[]{}.productId | String | Your unique product reference number or identifier to the product record in your system. |
question[]{}.serviceId | String | Unique service reference identifier. |
question[]{}.visibleOnAnswerId | String | If this is a null value, then this question should always be visible to the customer. If this field does have a value, then this question should not appear until the corresponding answer{}.id has been selected by the customer. |
question[]{}.sortOrder | Integer | Sort order the question should appear in |
question[]{}.required | Boolean | Whether or not the question is required to be answered |
question[]{}.type | String | The type of question. See Question Types for all possible values. |
question[]{}.question | String | The question to be asked of the customer |
question[]{}.answer[] | Array | An array of Answer objects only applicable to BOOLEAN , RADIO , CHECKBOX , and DROPDOWN question types. |
question[]{}.answer[]{}.id | String | Unique identifer of the answer record for a particular question |
question[]{}.answer[]{}.answer | String | The answer option to be presented to the customer |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |