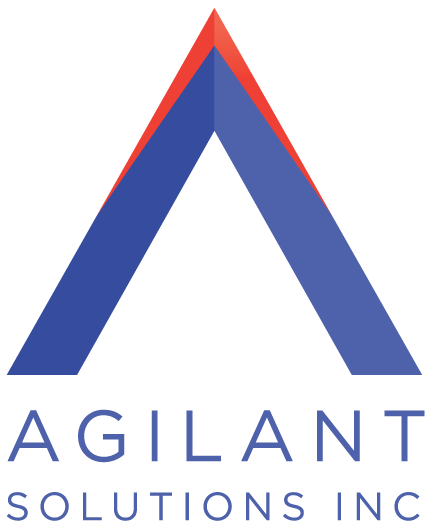
Overview
Introduction
This API implements functionality for managing accounts and services within our TOGa system through a RESTful HTTP transaction containing a JSON payload.
Endpoints
Beta/Test/Sandbox Environmenthttps://api.beta.agilantsolutions.com
Production Environmenthttps://api.agilantsolutions.com
Structure & Schema
- All requests and responses are formatted in JSON notation.
- Any whitespace in the request payload is disregarded. The JSON examples in this document are shown in a "pretty print" structure for easier legibility but JSON is not required to be in "pretty print" when performing the actual transactions.
- This API is intended for businesses-to-businesses (B2B) and must be implemented on your server-side. Requests to this API should never be sent from or processed by a user on the client-side.
- There are no data or structural differences between our beta and production environment. The only change you must make to initiate a request in production is that it must be sent to our production URL endpoint.
- All parameter names are case-sensitive and all values are handled with case sensitivity.
- Connection timeouts should be set to 15 seconds.
- Date and times in any inputs and outputs are formatted in ISO 8601 notation.
- For example:
1994-11-05
corresponds to November 5, 1994. - For example:
1994-11-05T08:15:30-05:00
corresponds to November 5, 1994, 8:15:30 am, US Eastern Standard Time. - For example:
08:15:30-05:00
corresponds to 8:15:30 am, US Eastern Standard Time.
Authentication & Authorization
- During the onboarding process, Agilant will provide you with two GUID keys which you are to store. One key is a public API key and the other key is a secret API key. You must ensure that the secret key is not disclosed anywhere in your code to the public.
- In the header of every transaction, you will need to provide a timestamp, your public key, and a signature of your transaction comprising of the timestamp field signed by your secret API key using the HMAC-SHA-256 cryptographic hash algorithm.
- The timestamp must be within 120 seconds (2 minutes) of the actual time or your transaction will be rejected. Therefore, you must ensure that your system time is maintained accurately within 2 minutes of the actual universal time.
Required Request Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Must be set to application/json |
Version | Integer | Must be exactly set to the value of 2 |
Timestamp | String | The current timestamp of your transaction in ISO 8601 notation. Example: 2019-01-02T17:34:52-05:00 |
Authorization | String | Concatenated string of your public API key, followed by a literal period (. ), followed by your signature. Your signature is your timestamp value, signed using your secret API key through the HMAC-SHA-256 cryptographic hash algorithm in a raw binary format and then Base64 encoded.Format: {publicKey}.{signatureOfTimestampUsingSecretKey} |
Sample Authentication Request Headers
Content-Type: application/json
Version: 2
Timestamp: 2019-01-02T17:34:52-05:00
Authorization: 1111AAAA-22BB-33CC-44DD-555555EEEEEE.Omjruf/UNd+rKEbjobxJzky84h6XOE9o9jz6/IKqc7Q=
Sample Authentication Request Headers
See the sample request using the sample information below.
- Using Sample Timestamp of:
2019-01-02T17:34:52-05:00
- Using Sample Public API Key of:
1111AAAA-22BB-33CC-44DD-555555EEEEEE
- Using Sample Secret API Key of:
EEEE5555-DD44-CC33-BB22-AAAAAA111111
Sample PHP Code for Assembling Request Headers
<?php
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
'Version: 2',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
?>
Responses
- All responses include two fields called
success
anderror
. - The
success
field is a boolean data type which will returntrue
orfalse
for whether or not the transaction was successful. - The
error
field will contain an intelligible string of the error details if one occurred or will be anull
value if no error occurred. - Additionally, all transactions will respond with the following codes if they are successful:
200 OK
for allGET
,PUT
, andDELETE
requests.201 CREATED
for allPOST
requests.- When determining for whether or not the transaction was successful, you should check that the HTTP response code is
200 OK
or201 CREATED
and/or check that thesuccess
value is set totrue
.
Sample Response Headers
Content-Type: application/json
Version: 2
Content-Length: 354
Response Headers for Every Transaction
Header Field | Type | Description |
---|---|---|
Content-Type | String | Will always be set to application/json |
Version | Integer | Version of the API which processed the request. Will be set to the value of 2 . |
Content-Length | Integer | Length of the response payload in bytes. |
Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
401 UNAUTHORIZED | Authentication or authorization failed. | Review the returned errors and correct the issue. If the problem persists, you may not be permitted to perform this API call. |
403 FORBIDDEN | Access was denied when trying to retrieve data for a record. | Ensure you have proper authorization to access the record. |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
405 METHOD NOT ALLOWED | The requested action is not supported. | Ensure that you are calling a valid API method and using the correct method and route. |
408 REQUEST TIMEOUT | A connection error was encountered and the request may or may not have completed successfully. | Retry the transaction and/or perform other GET requests to check if the previous request succeeded. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
500 INTERNAL SERVER ERRROR | An unexpected internal server error was encountered. | Contact the administrator. Further analysis and debugging may need to be performed. |
502 BAD GATEWAY | A temporary network issue occurred when receiving the request. This is rare and typically caused by a very temporary network issue which should resolve within a few seconds. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
504 GATEWAY TIMEOUT | A network issue occurred when receiving the request. | Retry the transaction or try again later. If the problem persists, please contact the administrator. |
PHP Example
<?php
$endpoint = 'https://api.beta.agilantsolutions.com'; // SANDBOX ENDPOINT
//$endpoint = 'https://api.agilantsolutions.com'; // PRODUCTION ENDPOINT
$publicApiKey = '1111AAAA-22BB-33CC-44DD-555555EEEEEE'; // Replace with your Public API Key
$secretApiKey = 'EEEE5555-DD44-CC33-BB22-AAAAAA111111'; // Replace with your Secret API Key
$method = 'GET';
$route = '/provider';
$payload = '{"providers":["C638C170-3EC1-4DF2-942C-1D1C3AD74FBC","16527A9E-4048-452CA8DF-605E1038A313"]}';
//////////////////////////////////////////////////
$url = ($endpoint . $route);
$timestamp = date('c', time());
$headers = [
'Content-Type: application/json',
('Timestamp: '.$timestamp),
('Authorization: '.$publicApiKey.'.'.base64_encode(hash_hmac('SHA256', $timestamp, $secretApiKey, true)))
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, $method);
curl_setopt($ch, CURLOPT_POSTFIELDS, $payload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$responseBody = curl_exec($ch);
$responseCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
echo 'responseCode=' . $responseCode . '<br>';
echo 'responseBody=' . $responseBody;
///////////////////////////////////////////////
/*
EXAMPLE OUTPUT:
responseCode=200
responseBody=
{
"success": true,
"error": null,
"providers": [
{
"providerId": "C638C170-3EC1-4DF2-942C-1D1C3AD74FBC",
"providerName": "Porch",
"avgRating": 4.3,
"reviews": [
{
"name": "Jane Doe",
"rating": 4.7,
"review": "I like this service very much!"
},
{
"name": "John Smith",
"rating": 3.5,
"review": "I was quite happy!"
}
]
},
{
"providerId": "16527A9E-4048-452C-A8DF-605E1038A313",
"providerName": "Hello Tech",
"avgRating": 3.1,
"reviews": [
{
"name": "Mary Doe",
"rating": 2.7,
"review": "I was not happy with this service.
},
{
"name": "Jim Dean",
"rating": 4.1,
"review": "This looks really nice!"
}
]
}
]
}
*/
Enumerated Lists
Account Status
Defines the account statuses.
Values
Value | Description |
---|---|
ACTIVE | Account services are active |
INACTIVE | Account services are inactive |
Service Status
Defines the service statuses.
Values
Value | Description |
---|---|
ACTIVE | Service is active |
INACTIVE | Service is inactive |
CANCELLED | Service has been cancelled |
Order Status
Defines the order statuses.
Values
Value | Description |
---|---|
QUOTE_CREATED | New quote record which is unpaid and unconfirmed |
QUOTE_INCOMPLETE | Quote which is missing required information |
QUOTE_AWAITING_DIAGNOSTIC | Quote which is awaiting results of a diagnostic |
QUOTE_DIAGNOSTIC_IN_PROGRESS | Quote which is currently undergoing a diagnostic |
QUOTE_DIAGNOSTIC_COMPLETE | Quote which has had a diagnostic completed |
QUOTE_DIAGNOSTIC_COMPLETE_FULL_SCAN_RECOMMENDED | Quote which has had a diagnostic completed but is recommending a full scan |
QUOTE_PAYMENT_PENDING | Quote which is ready and pending payment confirmation |
QUOTE_PAYMENT_ACCEPTED | Quote which is ready and a payment has been accepted |
ORDER_ISSUE_INCOMPLETE | Paid order which is missing required information |
ORDER_ISSUE_ACTION_NEEDED | Paid order which is pending a required action to be completed |
ORDER_SERVICE_PENDING | Paid order which has services pending |
ORDER_IN_PROGRESS | Paid order which has services currently being executed |
ORDER_COMPLETE | Paid order in which all services have been executed |
ORDER_CANCELLED | Paid order which was cancelled |
Order Type
Defines the order type.
Values
Value | Description |
---|---|
NEW | The order is for a new PC |
EXISTING | The order is for an existing PC |
Item Status
Defines the status of an item.
Values
Value | Description |
---|---|
CREATED | The item is created and unpaid |
PAID | The item is paid for |
ISSUE_INCOMPLETE | The item is missing required information |
ISSUE_ACTION_NEEDED | The item requires an action to be taken |
SERVICE_PENDING | A service appointment is pending |
IN_PROGRESS | A service appointment is in progress |
COMPLETE | The service on the item is completed |
CANCELLED | The service on the item has been cancelled |
Webhook Types
Defines the type of a webhook request
Values
Value | Description |
---|---|
ACCOUNT_STATUS_CHANGE | Occurs when an order status has changed. |
SERVICE_STATUS_CHANGE | Occurs when an order status has changed. |
ORDER_STATUS_CHANGE | Occurs when an order status has changed. |
ITEM_STATUS_CHANGE | Occurs when the status of an item changes. |
Data Objects
Address
Sample Address Object
{
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "XYZ Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
}
Represents an address object.
Fields
Field | Type | Description |
---|---|---|
firstName | String | Address first name |
lastName | String | Address last name |
businessName | String | Address business name |
address1 | String | Address line 1 |
address2 | String | Address line 2 |
city | String | Address city |
state | String | Address state |
zipCode | String | Address ZIP code |
Account
Sample Account Object
{
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company",
"createDate" : "2019-08-06",
"status" : "ACTIVE",
"service" : [
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"status" : "ACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
},
{
"lineNumber" : 2,
"skuNumber" : "IHIO-SMARTTV",
"status" : "INACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : null,
"renewalDate" : null,
"expirationDate" : null,
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "1234524456246246",
"invoiceNumber" : "3456745472",
"comment" : "Please call upon arrival"
}
]
}
Represents an account object.
Fields
Field | Type | Description |
---|---|---|
id | String | Your reference id referring to the account record in your system. |
firstName | String | First name of the account holder |
lastName | String | Last name of the account holder |
emailAddress | String | Email address of the account holder |
phoneNumber | String | Phone number of the account holder |
businessName | String | Business name of the account holder |
createDate | Date | Date the account was created |
status | String | The status of the account. See Account Status for all values. |
service[] | Array | Array of Service objects |
service[]{}.lineNumber | Integer | Line number of the service on the account |
service[]{}.skuNumber | String | SKU number of the service record |
service[]{}.status | String | The status of the service. See Service Status for all values. |
service[]{}.purchaseDate | Date | Date of purchase |
service[]{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. |
service[]{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . |
service[]{}.expirationDate | Date | Date the service will expire. |
service[]{}.storeNumber | String | Store number the service was sold in |
service[]{}.associateId | String | Associate ID of the salesperson who sold the service |
service[]{}.transactionId | String | Transaction ID of the receipt the service was purchased on |
service[]{}.invoiceNumber | String | Invoice number the service was purchased on |
service[]{}.comment | String | Notes/comments of the service |
Service
Sample Service Object
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"status" : "ACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
}
Represents a service object.
Fields
Field | Type | Description |
---|---|---|
lineNumber | Integer | Line number of the service on the account |
skuNumber | String | SKU number of the service record |
status | String | The status of the service. See Service Status for all values. |
purchaseDate | Date | Date of purchase |
cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. |
renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . |
expirationDate | Date | Date the service will expire. |
storeNumber | String | Store number the service was sold in |
associateId | String | Associate ID of the salesperson who sold the service |
transactionId | String | Transaction ID of the receipt the service was purchased on |
invoiceNumber | String | Invoice number the service was purchased on |
comment | String | Notes/comments of the service |
Order
Sample Order Object
{
"number" : "89023475",
"type" : "NEW",
"status" : "QUOTE_CREATED",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@abccompany.com",
"phoneNumber" : "893-555-4523",
"businessName" : "ABC Company",
"createdOn" : "2021-09-15T14:35:00-05:00",
"updatedOn" : "2021-09-15T14:35:00-05:00",
"paid" : true,
"vendorId" : "45626",
"loyaltyId" : "457215",
"storeNumber" : "6125",
"associateId" : "3456",
"billTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"shipTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"item" : [
{
"lineNumber" : 1,
"skuNumber" : "890345563",
"partNumber" : "ABC-12345",
"upcCode" : "23457892345789",
"status" : "PAID",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart TV Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
},
{
"lineNumber" : 2,
"skuNumber" : "346346",
"partNumber" : "DEF-56756",
"upcCode" : "38924579458",
"status" : "COMPLETE",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart Doorbell Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
}
]
}
Represents an order object.
Fields
Field | Type | Description |
---|---|---|
number | String | Unique order number reference |
type | String | The type of order. See Order Type for all values. |
status | String | The current status of the order. See Order Status for all values. |
firstName | String | First name of the order holder |
lastName | String | Last name of the order holder |
emailAddress | String | Email address of the order holder |
phoneNumber | String | Phone number of the order holder |
businessName | String | Business name of the order holder |
createdOn | Date/Time | Date/time the order was created |
updatedOn | Date/Time | Date/time the order was created |
paid | Boolean | Whether or not ALL items have been for |
vendorId | String | Internal vendor identifier |
loyaltyId | String | Loyalty ID reference identifier |
storeNumber | String | Store number where the order was created |
associateId | String | Associate ID who initiated the order |
billTo{} | Address | Billing address information |
billTo{}.firstName | String | Address first name |
billTo{}.lastName | String | Address last name |
billTo{}.businessName | String | Address business name |
billTo{}.address1 | String | Address line 1 |
billTo{}.address2 | String | Address line 2 |
billTo{}.city | String | Address city |
billTo{}.state | String | Address state |
billTo{}.zipCode | String | Address ZIP code |
shipTo{} | Address | Shipping address information |
shipTo{}.firstName | String | Address first name |
shipTo{}.lastName | String | Address last name |
shipTo{}.businessName | String | Address business name |
shipTo{}.address1 | String | Address line 1 |
shipTo{}.address2 | String | Address line 2 |
shipTo{}.city | String | Address city |
shipTo{}.state | String | Address state |
shipTo{}.zipCode | String | Address ZIP code |
item[] | Array | Array of Item objects on the order. |
item[]{}.lineNumber | Integer | Line number of the item on the order |
item[]{}.skuNumber | String | SKU number of the item on the order |
item[]{}.partNumber | String | Manufacturer's part number of the item |
item[]{}.upcCode | String | UPC code of the item |
item[]{}.status | String | Current status of the item. See Item Status for all values. |
item[]{}.quantity | Integer | Unit quantity of the item |
item[]{}.paid | Boolean | Whether or not the item has been paid for |
item[]{}.returned | Boolean | Whether or not the item has been returned |
item[]{}.returnable | Boolean | Whether or not the item is returnable |
item[]{}.voidable | Boolean | Whether or not the item is voidable |
item[]{}.discountable | Boolean | Whether or not the item is discountable |
item[]{}.taxable | Boolean | Whether or not the item is taxable |
item[]{}.isSubscription | Boolean | Whether or not the item is a recurring subscription |
item[]{}.createdOn | Date/Time | Date/time the item was created on |
item[]{}.paidOn | Date/Time | Date/time the item was paid for |
item[]{}.title | String | Title of the item |
item[]{}.shortDescription | String | Short description of the item |
item[]{}.longDescription | String | Long description of the item |
item[]{}.termsAndConditions | String | Item-level terms & conditions |
Item
Sample Item Object
{
"lineNumber" : 1,
"skuNumber" : "890345563",
"partNumber" : "ABC-12345",
"upcCode" : "23457892345789",
"status" : "PAID",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart TV Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
}
Represents an item object.
Fields
Field | Type | Description |
---|---|---|
lineNumber | Integer | Line number of the item on the order |
skuNumber | String | SKU number of the item on the order |
partNumber | String | Manufacturer's part number of the item |
upcCode | String | UPC code of the item |
status | String | Current status of the item. See Item Status for all values. |
quantity | Integer | Unit quantity of the item |
paid | Boolean | Whether or not the item has been paid for |
returned | Boolean | Whether or not the item has been returned |
returnable | Boolean | Whether or not the item is returnable |
voidable | Boolean | Whether or not the item is voidable |
discountable | Boolean | Whether or not the item is discountable |
taxable | Boolean | Whether or not the item is taxable |
isSubscription | Boolean | Whether or not the item is a recurring subscription |
createdOn | Date/Time | Date/time the item was created on |
paidOn | Date/Time | Date/time the item was paid for |
title | String | Title of the item |
shortDescription | String | Short description of the item |
longDescription | String | Long description of the item |
termsAndConditions | String | Item-level terms & conditions |
Accounts
Retrieve an AccountGET /account/{account.id}
Use this request to retrieve an account and view its status or information
Sample Request
GET /account/23526813
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null,
"account" : {
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company",
"createDate" : "2019-08-06",
"status" : "ACTIVE",
"service" : [
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"status" : "ACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
},
{
"lineNumber" : 2,
"skuNumber" : "IHIO-SMARTTV",
"status" : "INACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : null,
"renewalDate" : null,
"expirationDate" : null,
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "1234524456246246",
"invoiceNumber" : "3456745472",
"comment" : "Please call upon arrival"
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
account{} | Account | Contains the returned account object. |
account{}.id | String | Your reference id referring to the account record in your system. |
account{}.firstName | String | First name of the account holder |
account{}.lastName | String | Last name of the account holder |
account{}.emailAddress | String | Email address of the account holder |
account{}.phoneNumber | String | Phone number of the account holder |
account{}.businessName | String | Business name of the account holder |
account{}.createDate | Date | Date the account was created |
account{}.status | String | The status of the account. See Account Status for all values. |
account{}.service[] | Array | Array of Service objects |
account{}.service[]{}.lineNumber | Integer | Line number of the service on the account |
account{}.service[]{}.skuNumber | String | SKU number of the service record |
account{}.service[]{}.status | String | The status of the service. See Service Status for all values. |
account{}.service[]{}.purchaseDate | Date | Date of purchase |
account{}.service[]{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. |
account{}.service[]{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . |
account{}.service[]{}.expirationDate | Date | Date the service will expire. |
account{}.service[]{}.storeNumber | String | Store number the service was sold in |
account{}.service[]{}.associateId | String | Associate ID of the salesperson who sold the service |
account{}.service[]{}.transactionId | String | Transaction ID of the receipt the service was purchased on |
account{}.service[]{}.invoiceNumber | String | Invoice number the service was purchased on |
account{}.service[]{}.comment | String | Notes/comments of the service |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Create an AccountPOST /account
Use this request to create one or many accounts.
Sample Request
POST /account
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"account" : [
{
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company",
"service" : [
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
},
{
"lineNumber" : 2,
"skuNumber" : "IHIO-SMARTTV",
"purchaseDate" : "2020-01-01",
"cancellationDate" : null,
"renewalDate" : null,
"expirationDate" : null,
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "1234524456246246",
"invoiceNumber" : "3456745472",
"comment" : "Please call upon arrival"
}
]
}
]
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
account[] | Array | An array of Account objects. | Yes |
account[]{}.id | String | Your reference id referring to the account record in your system. | Yes |
account[]{}.firstName | String | First name of the account holder | Yes |
account[]{}.lastName | String | Last name of the account holder | Yes |
account[]{}.emailAddress | String | Email address of the account holder | Yes |
account[]{}.phoneNumber | String | Phone number of the account holder | No |
account[]{}.businessName | String | Business name of the account holder | No |
account[]{}.service[] | Array | Array of Service objects | No |
account[]{}.service[]{}.lineNumber | Integer | Line number of the service on the account | No |
account[]{}.service[]{}.skuNumber | String | SKU number of the service record | Yes |
account[]{}.service[]{}.purchaseDate | Date | Date of purchase | Yes |
account[]{}.service[]{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. | No |
account[]{}.service[]{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . | No |
account[]{}.service[]{}.expirationDate | Date | Date the service will expire. | No |
account[]{}.service[]{}.storeNumber | String | Store number the service was sold in | No |
account[]{}.service[]{}.associateId | String | Associate ID of the salesperson who sold the service | No |
account[]{}.service[]{}.transactionId | String | Transaction ID of the receipt the service was purchased on | No |
account[]{}.service[]{}.invoiceNumber | String | Invoice number the service was purchased on | No |
account[]{}.service[]{}.comment | String | Notes/comments of the service | No |
Sample Response
HTTP/1.1 201 CREATED
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
400 BAD REQUEST | A logic error was encountered or the request was missing a required parameter. | Review the returned errors and correct the issue. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Modify an AccountPUT /account/{account.id}
Use this request to modify an account in the system.
Sample Request
PUT /account/23526813
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"account" : {
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company"
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
account{} | Account | The account to modify | Yes |
account{}.id | String | Your reference id referring to the account record in your system. | No |
account{}.firstName | String | First name of the account holder | No |
account{}.lastName | String | Last name of the account holder | No |
account{}.emailAddress | String | Email address of the account holder | No |
account{}.phoneNumber | String | Phone number of the account holder | No |
account{}.businessName | String | Business name of the account holder | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Delete an AccountDELETE /account/{account.id}
Use this request to delete an account and cancel any outstanding subscriptions
Sample Request
DELETE /account/23526813
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Retrieve Agent LinkGET /account/{account.id}/link/agent
Use this request to retrieve the unique agent download link for the account.
Sample Request
GET /account/23526813/link/agent
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null,
"link" : "https://test.agentlink.com/ABCDEFG12345"
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
link | String | Contains the returned download link string. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Services
Create a ServicePOST /account/{account.id}/service
Use this request to add one or more new services to an account
Sample Request
POST /account/23526813/service
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"service" : {
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
service{} | Service | The service to add to the account | Yes |
service{}.skuNumber | String | SKU number of the service record | Yes |
service{}.purchaseDate | Date | Date of purchase | Yes |
service{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. | No |
service{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . | No |
service{}.expirationDate | Date | Date the service will expire. | No |
service{}.storeNumber | String | Store number the service was sold in | No |
service{}.associateId | String | Associate ID of the salesperson who sold the service | No |
service{}.transactionId | String | Transaction ID of the receipt the service was purchased on | No |
service{}.invoiceNumber | String | Invoice number the service was purchased on | No |
service{}.comment | String | Notes/comments of the service | No |
No input/request parameters are accepted.
Sample Response
HTTP/1.1 201 CREATED
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
201 CREATED | The record was successfully created. (Applicable only to POST requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
422 UNPROCESSABLE ENTITY | The request structure is correct and the server understands the request but is unable to process the transaction. This typically can occur in a POST request if the record being created or changed already exists. | Ensure you are not trying to create the same record that was already processed in a previous transaction. |
Modify a ServicePUT /account/{account.id}/service/{service.skuNumber}
Use this request to modify a service on an account.
Sample Request
PUT /account/23526813/service/PREM-SECURITY
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"service" : {
"lineNumber" : 1,
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
service{} | Service | The service to modify | Yes |
service{}.lineNumber | Integer | Line number of the service on the account | Yes |
service{}.purchaseDate | Date | Date of purchase | No |
service{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. | No |
service{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . | No |
service{}.expirationDate | Date | Date the service will expire. | No |
service{}.storeNumber | String | Store number the service was sold in | No |
service{}.associateId | String | Associate ID of the salesperson who sold the service | No |
service{}.transactionId | String | Transaction ID of the receipt the service was purchased on | No |
service{}.invoiceNumber | String | Invoice number the service was purchased on | No |
service{}.comment | String | Notes/comments of the service | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Delete a ServiceDELETE /account/{account.id}/service/{service.skuNumber}
Use this request to remove a service from an account.
Sample Request
DELETE /account/23526813/service/PREM-SECURITY
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"lineNumber" : 1
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
lineNumber | Integer | The line number of the service to make the change upon. *Required for multiple services of the same SKU number. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Orders
Retrieve an OrderGET /order/{order.number || order.transactionId}
Use this request to retrieve order details of an order number or transaction ID. This call should be used to check an order status or get its details.
Sample Request
GET /order/89023475
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
Input/Request Parameters
No input/request parameters are accepted.
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null,
"order" : {
"number" : "89023475",
"type" : "NEW",
"status" : "QUOTE_CREATED",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@abccompany.com",
"phoneNumber" : "893-555-4523",
"businessName" : "ABC Company",
"createdOn" : "2021-09-15T14:35:00-05:00",
"updatedOn" : "2021-09-15T14:35:00-05:00",
"paid" : true,
"vendorId" : "45626",
"loyaltyId" : "457215",
"storeNumber" : "6125",
"associateId" : "3456",
"billTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"shipTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"item" : [
{
"lineNumber" : 1,
"skuNumber" : "890345563",
"partNumber" : "ABC-12345",
"upcCode" : "23457892345789",
"status" : "PAID",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart TV Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
},
{
"lineNumber" : 2,
"skuNumber" : "346346",
"partNumber" : "DEF-56756",
"upcCode" : "38924579458",
"status" : "COMPLETE",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart Doorbell Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
}
]
}
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
order{} | Order | Contains the returned order details. |
order{}.number | String | Unique order number reference |
order{}.type | String | The type of order. See Order Type for all values. |
order{}.status | String | The current status of the order. See Order Status for all values. |
order{}.firstName | String | First name of the order holder |
order{}.lastName | String | Last name of the order holder |
order{}.emailAddress | String | Email address of the order holder |
order{}.phoneNumber | String | Phone number of the order holder |
order{}.businessName | String | Business name of the order holder |
order{}.createdOn | Date/Time | Date/time the order was created |
order{}.updatedOn | Date/Time | Date/time the order was created |
order{}.paid | Boolean | Whether or not ALL items have been for |
order{}.vendorId | String | Internal vendor identifier |
order{}.loyaltyId | String | Loyalty ID reference identifier |
order{}.storeNumber | String | Store number where the order was created |
order{}.associateId | String | Associate ID who initiated the order |
order{}.billTo{} | Address | Billing address information |
order{}.billTo{}.firstName | String | Address first name |
order{}.billTo{}.lastName | String | Address last name |
order{}.billTo{}.businessName | String | Address business name |
order{}.billTo{}.address1 | String | Address line 1 |
order{}.billTo{}.address2 | String | Address line 2 |
order{}.billTo{}.city | String | Address city |
order{}.billTo{}.state | String | Address state |
order{}.billTo{}.zipCode | String | Address ZIP code |
order{}.shipTo{} | Address | Shipping address information |
order{}.shipTo{}.firstName | String | Address first name |
order{}.shipTo{}.lastName | String | Address last name |
order{}.shipTo{}.businessName | String | Address business name |
order{}.shipTo{}.address1 | String | Address line 1 |
order{}.shipTo{}.address2 | String | Address line 2 |
order{}.shipTo{}.city | String | Address city |
order{}.shipTo{}.state | String | Address state |
order{}.shipTo{}.zipCode | String | Address ZIP code |
order{}.item[] | Array | Array of Item objects on the order. |
order{}.item[]{}.lineNumber | Integer | Line number of the item on the order |
order{}.item[]{}.skuNumber | String | SKU number of the item on the order |
order{}.item[]{}.partNumber | String | Manufacturer's part number of the item |
order{}.item[]{}.upcCode | String | UPC code of the item |
order{}.item[]{}.status | String | Current status of the item. See Item Status for all values. |
order{}.item[]{}.quantity | Integer | Unit quantity of the item |
order{}.item[]{}.paid | Boolean | Whether or not the item has been paid for |
order{}.item[]{}.returned | Boolean | Whether or not the item has been returned |
order{}.item[]{}.returnable | Boolean | Whether or not the item is returnable |
order{}.item[]{}.voidable | Boolean | Whether or not the item is voidable |
order{}.item[]{}.discountable | Boolean | Whether or not the item is discountable |
order{}.item[]{}.taxable | Boolean | Whether or not the item is taxable |
order{}.item[]{}.isSubscription | Boolean | Whether or not the item is a recurring subscription |
order{}.item[]{}.createdOn | Date/Time | Date/time the item was created on |
order{}.item[]{}.paidOn | Date/Time | Date/time the item was paid for |
order{}.item[]{}.title | String | Title of the item |
order{}.item[]{}.shortDescription | String | Short description of the item |
order{}.item[]{}.longDescription | String | Long description of the item |
order{}.item[]{}.termsAndConditions | String | Item-level terms & conditions |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Modify an OrderPUT /order/{order.number || order.transactionId}
Use this request to update or change order details including payment confirmation.
Sample Request
PUT /order/89023475
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
{
"order" : {
"number" : "89023475",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@abccompany.com",
"phoneNumber" : "893-555-4523",
"businessName" : "ABC Company",
"loyaltyId" : "457215",
"storeNumber" : "6125",
"associateId" : "3456",
"billTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"shipTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"item" : [
{
"lineNumber" : 1,
"quantity" : 1,
"paid" : true,
"returned" : false
},
{
"lineNumber" : 2,
"quantity" : 1,
"paid" : true,
"returned" : false
}
]
}
}
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
order{} | Order | The order object to modify. | Yes |
order{}.number | String | Unique order number reference | No |
order{}.firstName | String | First name of the order holder | No |
order{}.lastName | String | Last name of the order holder | No |
order{}.emailAddress | String | Email address of the order holder | No |
order{}.phoneNumber | String | Phone number of the order holder | No |
order{}.businessName | String | Business name of the order holder | No |
order{}.loyaltyId | String | Loyalty ID reference identifier | No |
order{}.storeNumber | String | Store number where the order was created | No |
order{}.associateId | String | Associate ID who initiated the order | No |
order{}.billTo{} | Address | Billing address information | No |
order{}.billTo{}.firstName | String | Address first name | No |
order{}.billTo{}.lastName | String | Address last name | No |
order{}.billTo{}.businessName | String | Address business name | No |
order{}.billTo{}.address1 | String | Address line 1 | No |
order{}.billTo{}.address2 | String | Address line 2 | No |
order{}.billTo{}.city | String | Address city | No |
order{}.billTo{}.state | String | Address state | No |
order{}.billTo{}.zipCode | String | Address ZIP code | No |
order{}.shipTo{} | Address | Shipping address information | No |
order{}.shipTo{}.firstName | String | Address first name | No |
order{}.shipTo{}.lastName | String | Address last name | No |
order{}.shipTo{}.businessName | String | Address business name | No |
order{}.shipTo{}.address1 | String | Address line 1 | No |
order{}.shipTo{}.address2 | String | Address line 2 | No |
order{}.shipTo{}.city | String | Address city | No |
order{}.shipTo{}.state | String | Address state | No |
order{}.shipTo{}.zipCode | String | Address ZIP code | No |
order{}.item[] | Array | Array of Item objects on the order. | No |
order{}.item[]{}.lineNumber | Integer | Line number of the item on the order | No |
order{}.item[]{}.quantity | Integer | Unit quantity of the item | No |
order{}.item[]{}.paid | Boolean | Whether or not the item has been paid for | No |
order{}.item[]{}.returned | Boolean | Whether or not the item has been returned | No |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Delete an Order ItemDELETE /order/{order.number || order.transactionId}/item/{item.skuNumber}
Use this request to remove an item.
Sample Request
DELETE /order/89023475
Content-Type: application/json
Version: 2
Timestamp: (See Authentication & Authorization)
Authorization: (See Authentication & Authorization)
[]
Input/Request Parameters
Field | Type | Description | Required |
---|---|---|---|
lineNumber | Integer | The line number of the service to delete. *Required for multiple services of the same SKU number. | Yes |
Sample Response
HTTP/1.1 200 OK
{
"success" : true,
"error" : null
}
Output/Response Parameters
Field | Type | Description |
---|---|---|
success | Boolean | Will return true or false for whether or not the transaction was successful. |
error | String | Intelligible string of the error that occurred. Will be null if no error occurred. |
Typical Response Codes
HTTP Code | Meaning | Required Action |
---|---|---|
200 OK | The transaction was successful. (Applicable to GET , PUT , and DELETE requests) | N/A |
404 NOT FOUND | The record could not be located. | Ensure you have requested a record that exists. |
Webhooks
If a webhook has been configured with your account, Agilant Solutions will automatically POST
a JSON request to your provided endpoint when an event occurs.
- If specific authentication requirements are needed, please let Agilant Solutions know and the security requirements can likely be accommodated.
- Note that your server responses will not be processed by Agilant Solutions. Therefore, there is no retry policy for webhooks if your server responds unsuccessfully.
Account Status ChangePOST {YourEndpoint}
This webhook will trigger when the status of an account changes and will POST
an up-to-date Account object containing the status and all other account details.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "ACCOUNT_STATUS_CHANGE",
"account" : {
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company",
"createDate" : "2019-08-06",
"status" : "ACTIVE",
"service" : [
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"status" : "ACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
},
{
"lineNumber" : 2,
"skuNumber" : "IHIO-SMARTTV",
"status" : "INACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : null,
"renewalDate" : null,
"expirationDate" : null,
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "1234524456246246",
"invoiceNumber" : "3456745472",
"comment" : "Please call upon arrival"
}
]
}
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly ACCOUNT_STATUS_CHANGE . See Webhook Types for all possible webhook values. |
account{} | Account | Contains the returned account details. |
account{}.id | String | Your reference id referring to the account record in your system. |
account{}.firstName | String | First name of the account holder |
account{}.lastName | String | Last name of the account holder |
account{}.emailAddress | String | Email address of the account holder |
account{}.phoneNumber | String | Phone number of the account holder |
account{}.businessName | String | Business name of the account holder |
account{}.createDate | Date | Date the account was created |
account{}.status | String | The status of the account. See Account Status for all values. |
account{}.service[] | Array | Array of Service objects |
account{}.service[]{}.lineNumber | Integer | Line number of the service on the account |
account{}.service[]{}.skuNumber | String | SKU number of the service record |
account{}.service[]{}.status | String | The status of the service. See Service Status for all values. |
account{}.service[]{}.purchaseDate | Date | Date of purchase |
account{}.service[]{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. |
account{}.service[]{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . |
account{}.service[]{}.expirationDate | Date | Date the service will expire. |
account{}.service[]{}.storeNumber | String | Store number the service was sold in |
account{}.service[]{}.associateId | String | Associate ID of the salesperson who sold the service |
account{}.service[]{}.transactionId | String | Transaction ID of the receipt the service was purchased on |
account{}.service[]{}.invoiceNumber | String | Invoice number the service was purchased on |
account{}.service[]{}.comment | String | Notes/comments of the service |
Service Status ChangePOST {YourEndpoint}
This webhook will trigger when the status of a service changes and will POST
an up-to-date Account object containing the status and all other account details.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "SERVICE_STATUS_CHANGE",
"account" : {
"id" : "23526813",
"firstName" : "Jane",
"lastName" : "Doe",
"emailAddress" : "jdoe@abccompany.com",
"phoneNumber" : "534-555-3467",
"businessName" : "ABC Company",
"createDate" : "2019-08-06",
"status" : "ACTIVE",
"service" : [
{
"lineNumber" : 1,
"skuNumber" : "PREM-SECURITY",
"status" : "ACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : "2021-07-01",
"renewalDate" : "2021-01-01",
"expirationDate" : "2020-12-31",
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "2345789245789187635",
"invoiceNumber" : "873452484",
"comment" : "Please call upon arrival"
},
{
"lineNumber" : 2,
"skuNumber" : "IHIO-SMARTTV",
"status" : "INACTIVE",
"purchaseDate" : "2020-01-01",
"cancellationDate" : null,
"renewalDate" : null,
"expirationDate" : null,
"storeNumber" : "12345",
"associateId" : "98765",
"transactionId" : "1234524456246246",
"invoiceNumber" : "3456745472",
"comment" : "Please call upon arrival"
}
]
}
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly SERVICE_STATUS_CHANGE . See Webhook Types for all possible webhook values. |
account{} | Account | Contains the returned account details. |
account{}.id | String | Your reference id referring to the account record in your system. |
account{}.firstName | String | First name of the account holder |
account{}.lastName | String | Last name of the account holder |
account{}.emailAddress | String | Email address of the account holder |
account{}.phoneNumber | String | Phone number of the account holder |
account{}.businessName | String | Business name of the account holder |
account{}.createDate | Date | Date the account was created |
account{}.status | String | The status of the account. See Account Status for all values. |
account{}.service[] | Array | Array of Service objects |
account{}.service[]{}.lineNumber | Integer | Line number of the service on the account |
account{}.service[]{}.skuNumber | String | SKU number of the service record |
account{}.service[]{}.status | String | The status of the service. See Service Status for all values. |
account{}.service[]{}.purchaseDate | Date | Date of purchase |
account{}.service[]{}.cancellationDate | Date | Cancellation date that the service will be cancelled on. If this is null , the service will not be cancelled. |
account{}.service[]{}.renewalDate | Date | Date the service will be renewed. If this is null , the service will not be renewed and will be expire on the expirationDate . |
account{}.service[]{}.expirationDate | Date | Date the service will expire. |
account{}.service[]{}.storeNumber | String | Store number the service was sold in |
account{}.service[]{}.associateId | String | Associate ID of the salesperson who sold the service |
account{}.service[]{}.transactionId | String | Transaction ID of the receipt the service was purchased on |
account{}.service[]{}.invoiceNumber | String | Invoice number the service was purchased on |
account{}.service[]{}.comment | String | Notes/comments of the service |
Order Status ChangePOST {YourEndpoint}
This webhook will trigger when an order status has changed and will POST
an up-to-date Order object containing the status and order details.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "ORDER_STATUS_CHANGE",
"order" : {
"number" : "89023475",
"type" : "NEW",
"status" : "QUOTE_CREATED",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@abccompany.com",
"phoneNumber" : "893-555-4523",
"businessName" : "ABC Company",
"createdOn" : "2021-09-15T14:35:00-05:00",
"updatedOn" : "2021-09-15T14:35:00-05:00",
"paid" : true,
"vendorId" : "45626",
"loyaltyId" : "457215",
"storeNumber" : "6125",
"associateId" : "3456",
"billTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"shipTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"item" : [
{
"lineNumber" : 1,
"skuNumber" : "890345563",
"partNumber" : "ABC-12345",
"upcCode" : "23457892345789",
"status" : "PAID",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart TV Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
},
{
"lineNumber" : 2,
"skuNumber" : "346346",
"partNumber" : "DEF-56756",
"upcCode" : "38924579458",
"status" : "COMPLETE",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart Doorbell Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
}
]
}
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly ORDER_STATUS_CHANGE . See Webhook Types for all possible webhook values. |
order{} | Order | Contains the returned order object. |
order{}.number | String | Unique order number reference |
order{}.type | String | The type of order. See Order Type for all values. |
order{}.status | String | The current status of the order. See Order Status for all values. |
order{}.firstName | String | First name of the order holder |
order{}.lastName | String | Last name of the order holder |
order{}.emailAddress | String | Email address of the order holder |
order{}.phoneNumber | String | Phone number of the order holder |
order{}.businessName | String | Business name of the order holder |
order{}.createdOn | Date/Time | Date/time the order was created |
order{}.updatedOn | Date/Time | Date/time the order was created |
order{}.paid | Boolean | Whether or not ALL items have been for |
order{}.vendorId | String | Internal vendor identifier |
order{}.loyaltyId | String | Loyalty ID reference identifier |
order{}.storeNumber | String | Store number where the order was created |
order{}.associateId | String | Associate ID who initiated the order |
order{}.billTo{} | Address | Billing address information |
order{}.billTo{}.firstName | String | Address first name |
order{}.billTo{}.lastName | String | Address last name |
order{}.billTo{}.businessName | String | Address business name |
order{}.billTo{}.address1 | String | Address line 1 |
order{}.billTo{}.address2 | String | Address line 2 |
order{}.billTo{}.city | String | Address city |
order{}.billTo{}.state | String | Address state |
order{}.billTo{}.zipCode | String | Address ZIP code |
order{}.shipTo{} | Address | Shipping address information |
order{}.shipTo{}.firstName | String | Address first name |
order{}.shipTo{}.lastName | String | Address last name |
order{}.shipTo{}.businessName | String | Address business name |
order{}.shipTo{}.address1 | String | Address line 1 |
order{}.shipTo{}.address2 | String | Address line 2 |
order{}.shipTo{}.city | String | Address city |
order{}.shipTo{}.state | String | Address state |
order{}.shipTo{}.zipCode | String | Address ZIP code |
order{}.item[] | Array | Array of Item objects on the order. |
order{}.item[]{}.lineNumber | Integer | Line number of the item on the order |
order{}.item[]{}.skuNumber | String | SKU number of the item on the order |
order{}.item[]{}.partNumber | String | Manufacturer's part number of the item |
order{}.item[]{}.upcCode | String | UPC code of the item |
order{}.item[]{}.status | String | Current status of the item. See Item Status for all values. |
order{}.item[]{}.quantity | Integer | Unit quantity of the item |
order{}.item[]{}.paid | Boolean | Whether or not the item has been paid for |
order{}.item[]{}.returned | Boolean | Whether or not the item has been returned |
order{}.item[]{}.returnable | Boolean | Whether or not the item is returnable |
order{}.item[]{}.voidable | Boolean | Whether or not the item is voidable |
order{}.item[]{}.discountable | Boolean | Whether or not the item is discountable |
order{}.item[]{}.taxable | Boolean | Whether or not the item is taxable |
order{}.item[]{}.isSubscription | Boolean | Whether or not the item is a recurring subscription |
order{}.item[]{}.createdOn | Date/Time | Date/time the item was created on |
order{}.item[]{}.paidOn | Date/Time | Date/time the item was paid for |
order{}.item[]{}.title | String | Title of the item |
order{}.item[]{}.shortDescription | String | Short description of the item |
order{}.item[]{}.longDescription | String | Long description of the item |
order{}.item[]{}.termsAndConditions | String | Item-level terms & conditions |
Item Status ChangePOST {YourEndpoint}
This webhook will trigger when the status of an item changes and will POST
an up-to-date Order object containing the status and all other order details.
Sample Request to {YourEndpoint}
POST {YourEndpoint}
Content-Type: application/json
{
"webhook" : "ITEM_STATUS_CHANGE",
"order" : {
"number" : "89023475",
"type" : "NEW",
"status" : "QUOTE_CREATED",
"firstName" : "John",
"lastName" : "Smith",
"emailAddress" : "jsmith@abccompany.com",
"phoneNumber" : "893-555-4523",
"businessName" : "ABC Company",
"createdOn" : "2021-09-15T14:35:00-05:00",
"updatedOn" : "2021-09-15T14:35:00-05:00",
"paid" : true,
"vendorId" : "45626",
"loyaltyId" : "457215",
"storeNumber" : "6125",
"associateId" : "3456",
"billTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"shipTo" : {
"firstName" : "John",
"lastName" : "Smith",
"businessName" : "ABC Company",
"address1" : "123 Test Street",
"address2" : "Suite 413",
"city" : "Testville",
"state" : "TN",
"zipCode" : "12345"
},
"item" : [
{
"lineNumber" : 1,
"skuNumber" : "890345563",
"partNumber" : "ABC-12345",
"upcCode" : "23457892345789",
"status" : "PAID",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart TV Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart TV.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
},
{
"lineNumber" : 2,
"skuNumber" : "346346",
"partNumber" : "DEF-56756",
"upcCode" : "38924579458",
"status" : "COMPLETE",
"quantity" : 1,
"paid" : true,
"returned" : false,
"returnable" : true,
"voidable" : true,
"discountable" : true,
"taxable" : true,
"isSubscription" : false,
"createdOn" : "2021-09-15T14:35:00-05:00",
"paidOn" : "2021-09-16T17:23:54-05:00",
"title" : "In-Home Smart Doorbell Installation",
"shortDescription" : "A qualified and experienced technician will come to your house to install your smart doorbell.",
"longDescription" : "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec fermentum vehicula nisl, sed volutpat tellus sollicitudin id. Ut id risus lobortis, molestie neque eu, dictum metus.",
"termsAndConditions" : "Morbi dignissim augue massa, eget vestibulum erat molestie nec. Donec at lorem at lacus mollis condimentum bibendum non enim. Curabitur eu odio at dolor feugiat aliquet. Donec non ante faucibus, hendrerit felis eget, vulputate arcu."
}
]
}
}
Request Parameters to {YourEndpoint}
Field | Type | Description |
---|---|---|
webhook | String | Will be exactly ITEM_STATUS_CHANGE . See Webhook Types for all possible webhook values. |
order{} | Order | Contains the returned order object. |
order{}.number | String | Unique order number reference |
order{}.type | String | The type of order. See Order Type for all values. |
order{}.status | String | The current status of the order. See Order Status for all values. |
order{}.firstName | String | First name of the order holder |
order{}.lastName | String | Last name of the order holder |
order{}.emailAddress | String | Email address of the order holder |
order{}.phoneNumber | String | Phone number of the order holder |
order{}.businessName | String | Business name of the order holder |
order{}.createdOn | Date/Time | Date/time the order was created |
order{}.updatedOn | Date/Time | Date/time the order was created |
order{}.paid | Boolean | Whether or not ALL items have been for |
order{}.vendorId | String | Internal vendor identifier |
order{}.loyaltyId | String | Loyalty ID reference identifier |
order{}.storeNumber | String | Store number where the order was created |
order{}.associateId | String | Associate ID who initiated the order |
order{}.billTo{} | Address | Billing address information |
order{}.billTo{}.firstName | String | Address first name |
order{}.billTo{}.lastName | String | Address last name |
order{}.billTo{}.businessName | String | Address business name |
order{}.billTo{}.address1 | String | Address line 1 |
order{}.billTo{}.address2 | String | Address line 2 |
order{}.billTo{}.city | String | Address city |
order{}.billTo{}.state | String | Address state |
order{}.billTo{}.zipCode | String | Address ZIP code |
order{}.shipTo{} | Address | Shipping address information |
order{}.shipTo{}.firstName | String | Address first name |
order{}.shipTo{}.lastName | String | Address last name |
order{}.shipTo{}.businessName | String | Address business name |
order{}.shipTo{}.address1 | String | Address line 1 |
order{}.shipTo{}.address2 | String | Address line 2 |
order{}.shipTo{}.city | String | Address city |
order{}.shipTo{}.state | String | Address state |
order{}.shipTo{}.zipCode | String | Address ZIP code |
order{}.item[] | Array | Array of Item objects on the order. |
order{}.item[]{}.lineNumber | Integer | Line number of the item on the order |
order{}.item[]{}.skuNumber | String | SKU number of the item on the order |
order{}.item[]{}.partNumber | String | Manufacturer's part number of the item |
order{}.item[]{}.upcCode | String | UPC code of the item |
order{}.item[]{}.status | String | Current status of the item. See Item Status for all values. |
order{}.item[]{}.quantity | Integer | Unit quantity of the item |
order{}.item[]{}.paid | Boolean | Whether or not the item has been paid for |
order{}.item[]{}.returned | Boolean | Whether or not the item has been returned |
order{}.item[]{}.returnable | Boolean | Whether or not the item is returnable |
order{}.item[]{}.voidable | Boolean | Whether or not the item is voidable |
order{}.item[]{}.discountable | Boolean | Whether or not the item is discountable |
order{}.item[]{}.taxable | Boolean | Whether or not the item is taxable |
order{}.item[]{}.isSubscription | Boolean | Whether or not the item is a recurring subscription |
order{}.item[]{}.createdOn | Date/Time | Date/time the item was created on |
order{}.item[]{}.paidOn | Date/Time | Date/time the item was paid for |
order{}.item[]{}.title | String | Title of the item |
order{}.item[]{}.shortDescription | String | Short description of the item |
order{}.item[]{}.longDescription | String | Long description of the item |
order{}.item[]{}.termsAndConditions | String | Item-level terms & conditions |